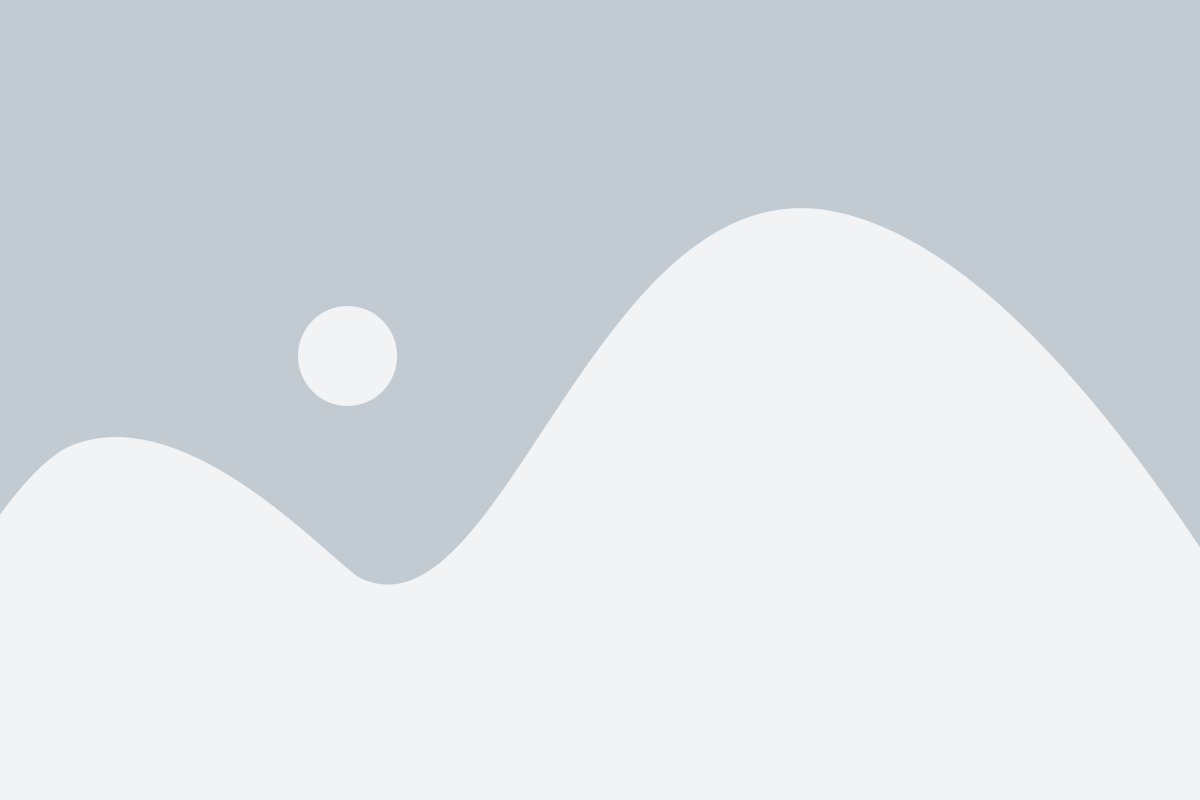
AI chatbots are transforming customer support, automating tasks, and enhancing user experiences. Building a chatbot in Python is easier than you might think, thanks to its rich ecosystem of libraries. In this guide, we’ll walk you through the process of creating a simple AI chatbot using Python and the NLTK and ChatterBot libraries.
Why Use Python for Chatbots?
Python is widely used for chatbot development because:
✅ Easy-to-Learn Syntax – Makes coding simple and efficient.
✅ Powerful Libraries – Offers NLTK, ChatterBot, and spaCy for natural language processing (NLP).
✅ Machine Learning Integration – Can be easily combined with deep learning frameworks like TensorFlow.
✅ Scalability – Can be deployed as a web-based or messaging chatbot.
Step 1: Install Required Libraries
Before building the chatbot, install the necessary Python libraries.
bash
pip install nltk chatterbot chatterbot_corpus
- NLTK (Natural Language Toolkit): For processing and understanding text.
- ChatterBot: For creating AI-based conversational responses.
- chatterbot_corpus: A collection of pre-trained chatbot data.
Step 2: Setting Up a Basic Chatbot
Let’s create a simple chatbot that responds based on predefined conversations.
Create a Python File (chatbot.py)
python
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
# Create a chatbot instance
chatbot = ChatBot(“SimpleBot”)
# Train the chatbot with the ChatterBot corpus
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train(“chatterbot.corpus.english”)
# Start a conversation loop
while True:
user_input = input(“You: “)
if user_input.lower() == “exit”:
print(“Chatbot: Goodbye!”)
break
response = chatbot.get_response(user_input)
print(“Chatbot:”, response)
How It Works:
- Creates a chatbot instance named SimpleBot.
- Uses ChatterBotCorpusTrainer to train the bot with a pre-built English dataset.
- Runs a loop where the user enters a message, and the bot responds.
- Ends conversation when the user types “exit”.
Run the script using:
bash
python chatbot.py
Step 3: Enhancing the Chatbot with Custom Responses
You can train your chatbot with custom responses to make it more intelligent.
Modify Training Data
Create a new training file (training_data.yml):
yaml
categories:
– greetings
conversations:
“Hello”
“Hi there!”
“How are you?”
“I’m good. How about you?”
“Goodbye”
“See you later!”
Now, modify the chatbot script to train with this dataset:
python
from chatterbot.trainers import ListTrainer
trainer = ListTrainer(chatbot)
trainer.train([
“Hello”,
“Hi there!”,
“How are you?”,
“I’m good. How about you?”,
“Goodbye”,
“See you later!”
])
Run the script again, and now the chatbot will recognize custom responses!
Step 4: Adding Natural Language Processing (NLP)
To make the chatbot smarter, integrate NLTK for NLP processing.
Install NLTK and Download Data
bash
pip install nltk
Incorporate NLP features like tokenization, stemming, and stop-word removal:
python
import nltk
from nltk.chat.util import Chat, reflections
pairs = [
[“hi|hello”, [“Hello!”, “Hi there!”]],
[“how are you?”, [“I’m fine, thank you!”, “I’m doing great!”]],
[“what is your name?”, [“I’m an AI chatbot!”]],
[“bye”, [“Goodbye!”, “See you later!”]]
]
chatbot = Chat(pairs, reflections)
while True:
user_input = input(“You: “)
if user_input.lower() == “exit”:
print(“Chatbot: Goodbye!”)
break
response = chatbot.respond(user_input)
print(“Chatbot:”, response)
How It Works:
- Uses predefined pairs to match user inputs.
- Uses regular expressions to detect greetings and common questions.
- Uses Chat() to map user input to appropriate responses.
Step 5: Deploying the Chatbot as a Web App
To make the chatbot accessible online, use Flask for a web-based chatbot.
Install Flask
bash
pip install flask
Create app.py for Web Chatbot
python
from flask import Flask, render_template, request
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
app = Flask(__name__)
chatbot = ChatBot(“WebBot”)
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train(“chatterbot.corpus.english”)
@app.route(“/”)
def home():
return render_template(“index.html”)
@app.route(“/get”)
def get_bot_response():
user_text = request.args.get(“msg”)
return str(chatbot.get_response(user_text))
if __name__ == “__main__”:
app.run(debug=True)
Create index.html (Simple Chat UI)
html
<!DOCTYPE html>
<html>
<head>
<title>Chatbot</title>
</head>
<body>
<h1>Chatbot</h1>
<input type=”text” id=”userInput”>
<button onclick=”sendMessage()”>Send</button>
<p id=”chatOutput”></p>
<script>
function sendMessage() {
let userInput = document.getElementById(“userInput”).value;
fetch(“/get?msg=” + userInput)
.then(response => response.text())
.then(data => {
document.getElementById(“chatOutput”).innerHTML = “Bot: ” + data;
});
}
</script>
</body>
</html>
Run the Flask app:
bash
python app.py
Visit http://127.0.0.1:5000/ in your browser to chat with your bot.
Step 6: Future Improvements
To enhance your chatbot, consider:
- Integrating AI/ML – Use deep learning models like GPT or Dialogflow.
- Connecting to APIs – Pull real-time data (e.g., weather, news).
- Deploying on Messaging Apps – Integrate with WhatsApp, Telegram, or Facebook Messenger.
Conclusion
Building a chatbot in Python is straightforward with libraries like ChatterBot, NLTK, and Flask. By following this guide, you’ve created a basic AI chatbot, trained it with custom responses, and even built a web-based chatbot.