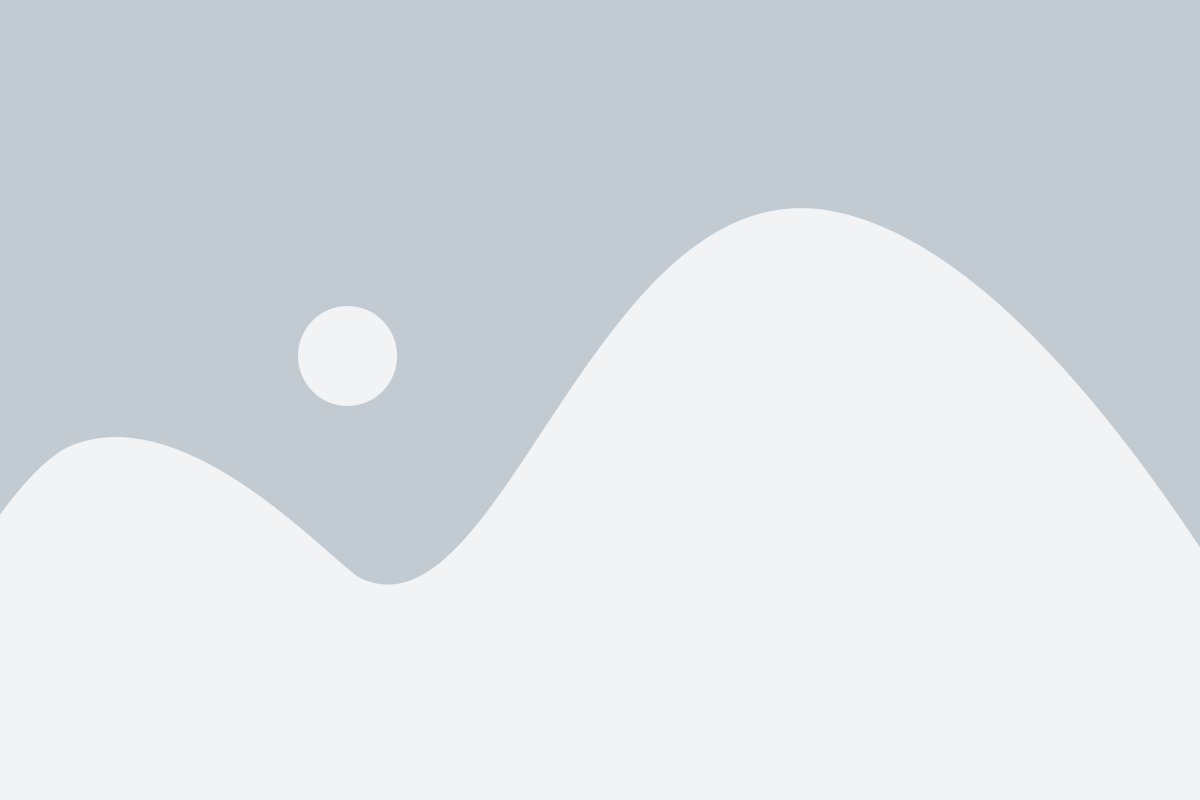
Data science is revolutionizing industries by turning raw data into meaningful insights. With Python as the preferred language for data science, beginners can easily dive into data analysis, visualization, and machine learning. Whether you are a student, analyst, or aspiring data scientist, this guide will help you get started with data science using Python.
Why Use Python for Data Science?
Python is widely used in data science because of:
✅ Easy-to-Learn Syntax – Python’s simplicity makes it beginner-friendly.
✅ Rich Ecosystem – Libraries like NumPy, Pandas, and Scikit-learn simplify tasks.
✅ Large Community Support – Access to extensive documentation and tutorials.
✅ Versatility – Used for data analysis, visualization, machine learning, and AI.
Step 1: Setting Up Your Python Environment
Before starting, install Python and the essential data science libraries.
Option 1: Using Anaconda (Recommended)
Anaconda is a distribution that includes Python and pre-installed data science libraries.
Installation:
- Download and install Anaconda.
- Open Jupyter Notebook or Spyder (IDE included in Anaconda).
Option 2: Using pip (Manual Installation)
If you prefer a lightweight setup, install Python and required libraries using pip:
bash
pip install numpy pandas matplotlib seaborn scikit-learn jupyterlab
Launch Jupyter Notebook for coding:
bash
jupyter notebook
Step 2: Understanding the Key Python Libraries
Python has powerful libraries for data science. Let’s explore some essential ones:
- NumPy – Numerical Computing
NumPy helps with array operations and numerical computations.
python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr.mean()) # Output: 3.0
- Pandas – Data Manipulation
Pandas is used to load, manipulate, and analyze data.
python
import pandas as pd
df = pd.read_csv(“data.csv”) # Load dataset
print(df.head()) # Display first 5 rows
- Matplotlib & Seaborn – Data Visualization
These libraries help visualize data trends and patterns.
python
import matplotlib.pyplot as plt
import seaborn as sns
sns.histplot(df[‘column_name’])
plt.show()
- Scikit-Learn – Machine Learning
Scikit-learn is used for predictive modeling.
python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
X_train, X_test, y_train, y_test = train_test_split(df[[‘feature’]], df[‘target’], test_size=0.2)
model = LinearRegression()
model.fit(X_train, y_train)
print(model.score(X_test, y_test)) # Model accuracy
Step 3: Data Collection and Cleaning
Data science starts with collecting and cleaning data. You can get data from CSV files, APIs, or databases.
Loading Data from CSV
python
df = pd.read_csv(“dataset.csv”)
print(df.info()) # Check data structure
Handling Missing Values
python
df.fillna(df.mean(), inplace=True) # Replace missing values with column mean
Removing Duplicates
python
df.drop_duplicates(inplace=True)
Step 4: Data Exploration and Visualization
Before building models, explore the data to find patterns.
Check Summary Statistics
python
print(df.describe()) # Statistical summary
Visualizing Data Trends
python
sns.pairplot(df)
plt.show()
Step 5: Building a Simple Machine Learning Model
Let’s build a basic linear regression model to predict house prices.
python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
X = df[[‘square_feet’]]
y = df[‘price’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
print(“Model Accuracy:”, model.score(X_test, y_test))
Step 6: Learning Advanced Topics
Once you are comfortable with the basics, explore:
- Deep Learning – Using TensorFlow and PyTorch.
- Natural Language Processing (NLP) – Text analysis with NLTK and SpaCy.
- Big Data – Working with Apache Spark.
- Deploying Models – Using Flask or FastAPI.
Conclusion
Vent academy provide Python is a powerful and beginner-friendly language for data science. By learning key libraries like Pandas, NumPy, and Scikit-learn, you can quickly start working on real-world data projects.