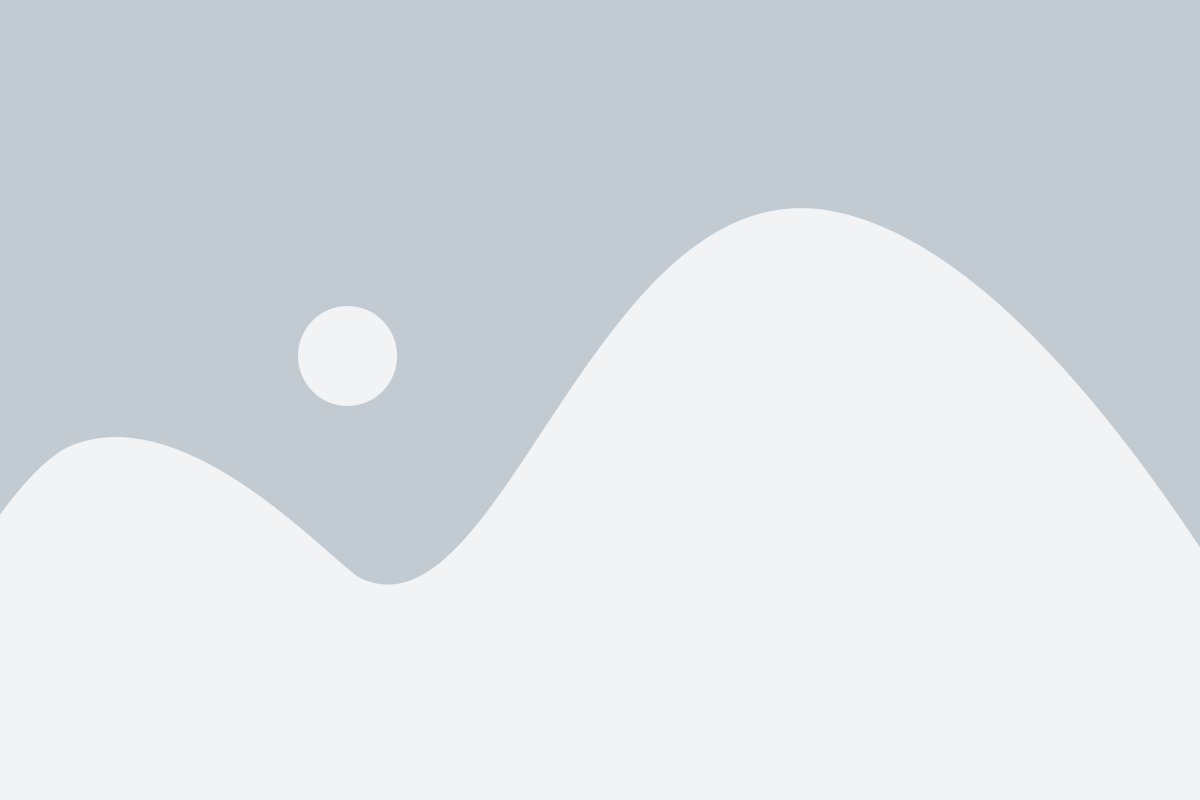
Introduction to Node.js
Node.js has dramatically transformed the web development landscape since its inception in 2009. Born from a desire to execute JavaScript server-side, Node.js has enabled developers to create highly scalable, event-driven applications using a language that was traditionally confined to client-side environments. This shift has opened new horizons for developers, bringing a fresh approach to building robust web applications.
The Rise of Node.js in Web Development
Node.js has surged in popularity, becoming a fundamental technology in modern web development. Its ascension can be attributed to its unique ability to unify client and server-side scripting, allowing developers to use a single language throughout the application stack. This capability reduces the learning curve, streamlines development, and facilitates code reuse. Node.js’s asynchronous architecture makes it a natural fit for building real-time applications, fueling its adoption across a wide range of industries.
What Makes Node.js Unique Among JavaScript Frameworks?
Node.js stands out among JavaScript frameworks due to its non-blocking, event-driven model. Unlike traditional server-side frameworks that rely on multi-threading, Node.js operates on a single-threaded event loop. This design allows it to handle a high volume of simultaneous connections with minimal overhead, making it ideal for scalable applications. Additionally, Node.js’s robust ecosystem, driven by npm (Node Package Manager), provides developers with a vast array of packages and libraries to extend its capabilities.
How Node.js Revolutionized the Server-Side Landscape
Node.js has revolutionized server-side development by introducing a lightweight, efficient, and scalable approach. Its event-driven nature enables developers to build applications that are responsive and capable of handling multiple concurrent requests without compromising performance. This revolution has led to a shift from traditional monolithic architectures to microservices and serverless models, further enhancing the flexibility and scalability of web applications.
Understanding Node.js Architecture
Node.js’s architecture is central to its success. At its core, Node.js relies on an event-driven programming model that allows it to manage a multitude of tasks simultaneously. This architecture empowers developers to build applications that can respond to events in real time, making Node.js the preferred choice for applications requiring low latency and high concurrency.
Event-Driven Programming: The Key to Node.js’s Power
Event-driven programming is the backbone of Node.js. In this model, the application listens for specific events, such as HTTP requests or file system changes, and triggers corresponding actions. This approach enables Node.js to handle multiple tasks concurrently without blocking the execution flow. The event loop processes events asynchronously, ensuring that the server remains responsive even under heavy loads.
Non-Blocking I/O: How It Works and Why It Matters
Non-blocking I/O is a fundamental aspect of Node.js. Unlike traditional server-side frameworks that rely on blocking operations, Node.js uses asynchronous calls to interact with external resources like databases and file systems. This non-blocking behavior allows Node.js to perform other tasks while waiting for I/O operations to complete, resulting in improved efficiency and reduced latency. This characteristic is especially crucial for real-time applications, where speed and responsiveness are paramount.
The Single-Threaded Event Loop Explained
The single-threaded event loop is the heart of Node.js’s architecture. Unlike multi-threaded frameworks that create a new thread for each request, Node.js operates on a single thread. This design might seem limiting, but the event loop ensures that Node.js can handle a high volume of requests by queuing events and processing them asynchronously. This approach allows Node.js to achieve high concurrency without the overhead associated with managing multiple threads, making it a powerful and efficient framework for server-side development.
Top Insider Tips for Writing Clean Node.js Code
Creating clean, maintainable code is essential for any successful Node.js project. By adopting best practices and following proven patterns, developers can build robust applications that are easy to understand and maintain. Here are some insider tips to help you write clean Node.js code.
Modular Code Structure: Keeping It Manageable
Modularity is key to maintaining a clean codebase. By breaking your application into smaller, reusable modules, you can reduce complexity and improve code organization. Modular code allows developers to work on specific components without affecting the rest of the application, making it easier to debug, test, and maintain. This approach also facilitates code reuse, allowing you to leverage existing modules in multiple parts of your application.
DRY (Don’t Repeat Yourself): Reducing Redundancy in Code
The “DRY” principle is a cornerstone of clean code. By avoiding redundancy and reusing code whenever possible, you can create a more concise and efficient codebase. In Node.js, this often involves creating utility functions and shared modules that encapsulate common functionality. By adhering to the DRY principle, you can reduce the likelihood of bugs and simplify the process of making changes to your code.
Implementing SOLID Principles for Better Design
The SOLID principles are a set of guidelines for creating robust and maintainable software design. In Node.js, implementing SOLID principles can help you create a more scalable and flexible codebase. These principles include:
Single Responsibility: Each module should have one responsibility.
Open/Closed: Code should be open for extension but closed for modification.
Liskov Substitution: Objects should be replaceable with their subtypes without affecting correctness.
Interface Segregation: Interfaces should be specific to clients, avoiding unnecessary dependencies.
Dependency Inversion: High-level modules should not depend on low-level modules; both should depend on abstractions.
By applying these principles, you can create a well-structured codebase that is easier to maintain and extend.
Proper Use of Promises and Async/Await to Handle Asynchronous Operations
Asynchronous operations are central to Node.js’s architecture, and handling them correctly is crucial for creating clean code. Promises and async/await are powerful tools for managing asynchronous tasks without falling into “callback hell.” Promises allow you to chain asynchronous operations, while async/await provides a more intuitive syntax for working with asynchronous code. Properly utilizing these constructs can help you create more readable and maintainable code while avoiding common pitfalls associated with asynchronous programming.
Essential Tools and Libraries for Node.js Developers
Node.js offers a rich ecosystem of tools and libraries designed to enhance developer productivity and streamline workflows. Leveraging these resources can significantly improve the efficiency of your Node.js projects.
Exploring the Rich Ecosystem of npm Packages
npm is the primary package manager for Node.js, providing access to thousands of libraries and modules. Whether you’re looking for utility functions, web frameworks, or specialized tools, npm has a package for nearly every use case. Exploring the npm ecosystem allows developers to find pre-built solutions to common problems, reducing development time and effort. Popular npm packages like Express, Lodash, and Moment.js are widely used in the Node.js community, offering a wealth of functionality and flexibility.
Debugging with Node-Inspect and Chrome DevTools
Debugging is a critical aspect of Node.js development. Tools like Node-Inspect and Chrome DevTools provide robust debugging capabilities, allowing you to set breakpoints, inspect variables, and step through code execution. Node-Inspect enables remote debugging, while Chrome DevTools offers a familiar interface for troubleshooting Node.js applications. By leveraging these debugging tools, developers can quickly identify and resolve issues, ensuring their code operates as expected.
Automating Tasks with Build Tools Like Webpack and Parcel
Build tools play a crucial role in modern web development, helping automate tasks like code bundling, transpilation, and minification. Webpack and Parcel are popular build tools that can be used with Node.js to streamline the development process. These tools facilitate asset bundling, allowing developers to combine JavaScript, CSS, and other resources into optimized bundles. This automation improves code efficiency and reduces the complexity of deploying Node.js applications.
Using Nodemon for Streamlined Development Workflow
Nodemon is an essential tool for Node.js developers, providing automatic server restarts when code changes. This feature allows developers to maintain a seamless workflow without manually restarting the server each time they make a change. By using Nodemon, you can save time and increase productivity during development, allowing you to focus on writing code instead of managing server restarts.
Common Mistakes and How to Avoid Them
Node.js development can be challenging, and common mistakes can lead to performance issues and unexpected bugs. By understanding these pitfalls and knowing how to avoid them, developers can create more reliable and stable applications.
The Dangers of Callback Hell and How to Prevent It
Callback hell is a notorious issue in Node.js development, arising when nested callbacks create convoluted and hard-to-read code. This pattern can lead to errors and make debugging difficult. To avoid callback hell, developers should use Promises or async/await to manage asynchronous operations. These constructs offer a cleaner and more maintainable approach to handling callbacks, reducing the risk of complex code structures.
Avoiding Memory Leaks in Long-Running Node.js Applications
Memory leaks can be detrimental to Node.js applications, leading to increased resource usage and reduced performance. In long-running applications, memory leaks can cause servers to crash or slow down over time. To prevent memory leaks, developers should ensure proper garbage collection and avoid holding onto unnecessary references. Using tools like Node.js’s built-in memory profiler, developers can identify potential memory leaks and address them before they become a problem.
Handling Errors Properly: Best Practices and Common Pitfalls
Error handling is crucial in Node.js applications, especially given the asynchronous nature of the framework. Failing to handle errors properly can result in unhandled exceptions and server crashes. Best practices for error handling in Node.js include using try/catch blocks, properly propagating errors through Promises, and implementing robust error logging. By following these practices, developers can ensure their applications remain stable and resilient.
Optimizing Performance in Node.js Applications
Optimizing performance is a top priority for Node.js developers, especially when building high-traffic applications. By implementing effective performance strategies, developers can ensure their applications remain responsive and scalable.
Strategies for Efficient Caching and Data Storage
Caching is a powerful technique for improving performance in Node.js applications. By storing frequently accessed data in memory, developers can reduce the need for repeated database queries or external API calls. Tools like Redis and Memcached offer in-memory caching solutions that can significantly boost performance. When implementing caching, developers should consider cache invalidation strategies to ensure data remains consistent and up-to-date.
Load Balancing and Clustering for High-Traffic Applications
Load balancing and clustering are essential strategies for handling high-traffic applications. Load balancing involves distributing incoming requests among multiple servers to prevent any single server from becoming overwhelmed. Tools like Nginx and HAProxy are commonly used for load balancing in Node.js environments. Clustering allows Node.js applications to utilize multiple cores by running several instances of the application concurrently. This approach can significantly increase concurrency and scalability.
Monitoring Performance with Tools Like New Relic and Datadog
Monitoring is crucial for maintaining optimal performance in Node.js applications. Tools like New Relic and Datadog provide comprehensive monitoring capabilities, allowing developers to track application performance and server health in real-time. These tools offer insights into response times, resource usage, and error rates, enabling developers to identify and address performance bottlenecks quickly. By implementing robust monitoring, developers can ensure their Node.js applications remain performant and responsive.
Security Best Practices in Node.js
Security is a critical aspect of Node.js development, and following best practices is essential for protecting applications from common threats. By understanding common vulnerabilities and implementing security-focused strategies, developers can build secure Node.js applications.
Identifying Common Security Vulnerabilities in Node.js
Node.js applications are susceptible to various security vulnerabilities, including SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Identifying and mitigating these vulnerabilities is crucial for ensuring the security of your application. SQL injection can occur when unsanitized user input is used in database queries, leading to unauthorized data access. XSS involves injecting malicious scripts into web pages, while CSRF exploits user sessions to perform unauthorized actions.
Protecting Against Injection Attacks, XSS, and CSRF
To protect against SQL injection, developers should always validate and sanitize user input before using it in database queries. Parameterized queries and ORM (Object-Relational Mapping) tools can help prevent injection attacks by abstracting the query-building process. To mitigate XSS, developers should ensure proper output encoding to prevent malicious scripts from executing on the client side. Implementing CSRF tokens and validating them on each request can help prevent CSRF attacks, ensuring only legitimate actions are performed.
Using Security Middleware Like Helmet to Enhance Application Security
Security middleware is a valuable asset in Node.js applications. Helmet, a popular security middleware, provides a collection of modules designed to enhance application security. These modules can set HTTP headers to prevent common vulnerabilities, enforce HTTPS, and mitigate the risk of clickjacking. By integrating Helmet into your Node.js application, you can bolster security and protect against a variety of threats.
Best Practices for Secure Authentication and Authorization
Authentication and authorization are critical components of a secure Node.js application. Best practices include using strong password hashing algorithms like bcrypt, implementing two-factor authentication, and securing JWT (JSON Web Token) tokens to prevent unauthorized access. Developers should also ensure that user roles and permissions are enforced throughout the application to maintain a secure environment. By following these best practices, developers can build robust authentication and authorization systems that protect user data and application integrity.
Advanced Topics and Future Trends in Node.js
Node.js continues to evolve, with new technologies and trends shaping the future of web development. Exploring advanced topics and emerging trends can help developers stay ahead of the curve and create innovative Node.js applications.
The Rise of Serverless Architectures in Node.js
Serverless architectures have gained popularity in recent years, offering a new way to build scalable applications without managing servers. In Node.js, serverless frameworks like AWS Lambda and Google Cloud Functions allow developers to write code that runs in response to specific events, such as HTTP requests or database changes. This approach eliminates the need for traditional server management, allowing developers to focus on application logic and reduce infrastructure costs.
GraphQL: A New Way to Build APIs with Node.js
GraphQL has emerged as a powerful alternative to traditional RESTful APIs. It provides a flexible query language that allows clients to request specific data from the server, reducing over-fetching and under-fetching. In Node.js, frameworks like Apollo Server enable developers to build GraphQL APIs with ease. GraphQL’s flexibility and efficiency make it an attractive option for modern web applications, allowing developers to create more dynamic and responsive interfaces.
Embracing TypeScript for Better Code Quality
TypeScript, a typed superset of JavaScript, has gained traction in the Node.js community for its ability to improve code quality and reliability. By adding static typing to JavaScript, TypeScript helps developers catch errors at compile-time, reducing runtime bugs and enhancing code maintainability. Embracing TypeScript in Node.js applications can lead to more robust code and improved developer productivity.
What’s Next for Node.js: Upcoming Features and Enhancements
Node.js continues to evolve, with ongoing development and new features on the horizon. The Node.js community is actively working on enhancing the framework’s performance, security, and scalability. Upcoming features and enhancements may include improved support for modern JavaScript syntax, enhanced concurrency capabilities, and additional security features. Staying informed about these developments can help developers leverage the latest advancements in Node.js and build cutting-edge web applications
Conclusion
Node.js has had a profound impact on web development, revolutionizing the way server-side applications are built and deployed. Its unique architecture, robust ecosystem, and scalability make it a powerful tool for modern developers. By leveraging Node.js, developers can create scalable, secure, and high-performance web applications that meet the demands of today’s digital landscape.
Encouraging Node.js adoption among developers is essential for the continued growth and innovation of the Node.js community. With a wealth of resources, tools, and libraries available, developers can tap into the collaborative spirit of the Node.js ecosystem to build exceptional applications. By embracing Node.js and staying informed about emerging trends, developers can continue to push the boundaries of what’s possible in the world of web development.