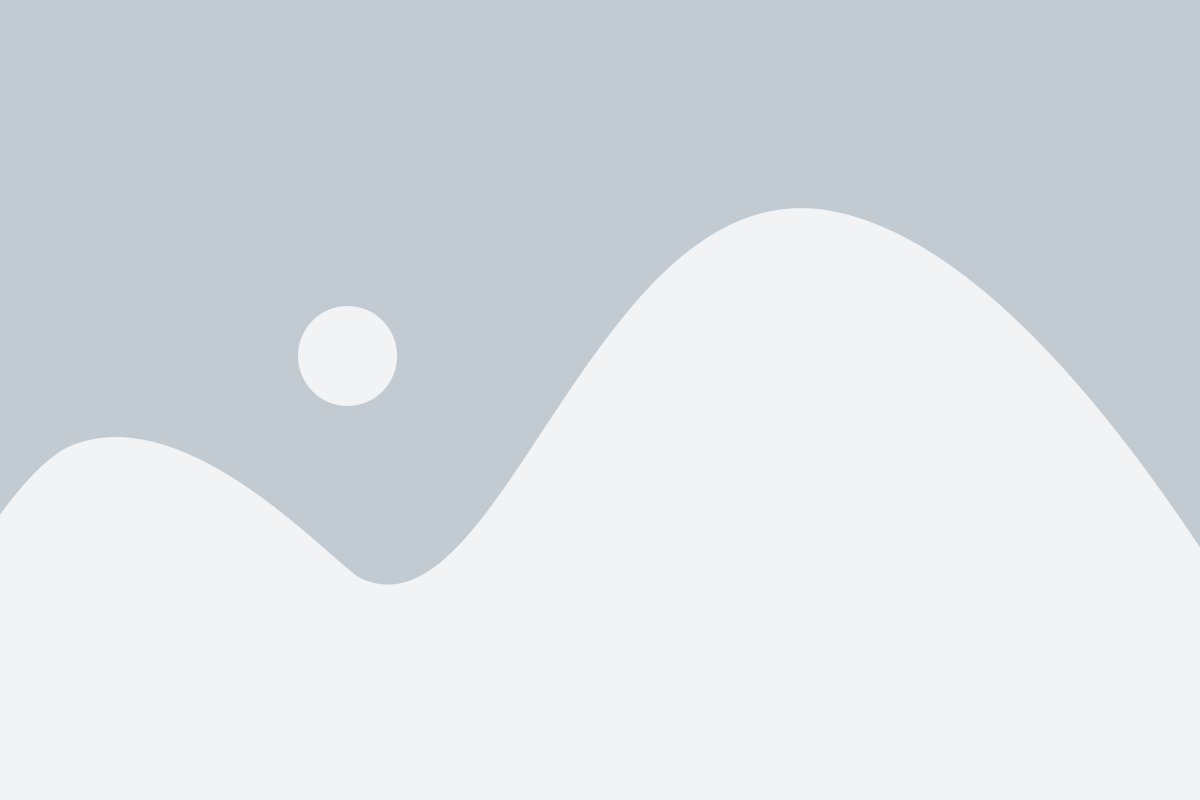
Introduction to Node.js
Node.js is a powerful, open-source, server-side runtime environment that has revolutionized the world of web development. Its ability to run JavaScript outside of the browser has paved the way for a new generation of server-side applications, microservices, and real-time communication. Before Node.js, JavaScript was confined to client-side scripting in web browsers. With Node.js, JavaScript has transcended this limitation, making it possible to build scalable, high-performance server-side applications using a language that’s already widely understood.
Understanding the Basics
To truly understand Node.js, one must dive into its core concepts, architecture, and the ecosystem that supports it.
What is Node.js?
Node.js is a runtime environment that allows developers to execute JavaScript on the server side. Built on Chrome’s V8 JavaScript engine, Node.js uses an event-driven, non-blocking I/O model, which makes it lightweight and efficient. This design allows Node.js to handle multiple concurrent operations, which is ideal for building scalable network applications.
Why Use Node.js?
Node.js has gained popularity for several reasons. Its event-driven architecture makes it perfect for applications that require real-time interaction, such as chat applications or online gaming. The non-blocking I/O model ensures high scalability, allowing Node.js to efficiently handle many connections simultaneously. Additionally, Node.js has a vibrant community and a rich ecosystem of packages, making it easy to find tools and libraries for various tasks.
Key Features and Advantages of Node.js
Node.js boasts numerous features that set it apart. Its asynchronous programming model allows for efficient handling of I/O operations without blocking other processes. This efficiency translates into improved performance and reduced latency, critical for web applications. Another key advantage is its cross-platform compatibility; Node.js runs on Windows, macOS, and Linux, making it accessible to a broad range of developers. The extensive collection of open-source packages via npm (Node Package Manager) further enhances its versatility.
Node.js Architecture and Environment
Node.js’s architecture plays a crucial role in its success. Understanding how it works is key to leveraging its capabilities.
Event-Driven Architecture
Node.js’s event-driven architecture is fundamental to its design. In this model, events are emitted when certain actions occur, such as a network connection or a file being read. Event listeners then respond to these events, executing the corresponding callback functions. This architecture allows Node.js to remain highly responsive even under heavy loads.
How the Event Loop Works
At the heart of Node.js’s event-driven architecture is the event loop. The event loop is responsible for continuously checking for new events and dispatching them to the appropriate event listeners. Unlike traditional server-side environments, where each request might spawn a new thread, Node.js handles all events in a single thread. This single-threaded approach might seem limiting, but the non-blocking nature of the event loop ensures that it can handle multiple operations concurrently without slowing down.
Asynchronous Programming in Node.js
Asynchronous programming is central to Node.js’s efficiency. In this paradigm, operations that might otherwise block execution—like reading from a database or making an HTTP request—are handled asynchronously. This approach allows the program to continue processing other tasks while waiting for the I/O operation to complete. Node.js achieves this with callbacks, promises, and the modern async/await syntax.
Callbacks, Promises, and Async/Await
Callbacks have been the traditional way to handle asynchronous operations in Node.js. A callback is a function passed as an argument to another function, which is then called when the asynchronous operation completes. However, callbacks can lead to “callback hell,” where nested callbacks create unreadable code. Promises were introduced to address this issue, allowing developers to chain asynchronous operations in a more linear fashion. The async/await syntax, introduced in ECMAScript 2017, further simplifies asynchronous code, allowing developers to write asynchronous operations as if they were synchronous.
Modules and Packages
Node.js’s modularity is one of its strengths. It allows developers to organize code into reusable pieces, improving maintainability and scalability.
Node.js Modules
Node.js modules are self-contained units of code that encapsulate specific functionality. This modular approach allows developers to build complex applications from smaller, manageable components. Node.js provides a robust system for creating, requiring, and exporting modules, making it easy to share code across different parts of an application or even across different projects.
Built-in Modules in Node.js
Node.js comes with a rich set of built-in modules that provide various functionalities. These modules cover everything from creating HTTP servers to managing file systems. Popular built-in modules include ‘fs’ for file system operations, ‘http’ for HTTP server creation, ‘os’ for interacting with the operating system, and ‘path’ for file path manipulations.
Creating and Using Custom Modules
In addition to the built-in modules, developers can create custom modules to encapsulate specific functionality. This approach promotes code reuse and makes it easier to maintain and test individual components. To create a custom module, developers define the desired functionality and export it for use in other parts of the application. The ‘require’ function is then used to import and use these custom modules.
Requiring and Exporting Modules
Node.js uses the CommonJS module system for requiring and exporting modules. The ‘module.exports’ object is used to define what a module exports, allowing other modules to import and use its functionality. The ‘require’ function is used to import modules, whether they are built-in, custom, or third-party. This modular approach simplifies code organization and promotes code reuse across different projects.
Working with NPM (Node Package Manager)
NPM is the lifeblood of the Node.js ecosystem. It provides a vast repository of packages, making it easy for developers to extend the functionality of their applications.
Introduction to NPM
Node Package Manager (NPM) is the default package manager for Node.js. It allows developers to install, update, and manage packages for their applications. With over a million packages available, NPM offers a solution for virtually every need, from building web servers to managing databases and integrating with third-party services.
Installing Packages with NPM
Installing packages with NPM is straightforward. Using simple commands like npm install <package-name>, developers can add packages to their projects. NPM also supports installing specific versions of packages and managing package dependencies with ease. This flexibility ensures that developers can maintain stable and consistent environments across different development and production environments.
Managing Project Dependencies
NPM manages project dependencies through the package.json file, which contains a list of all the packages required for a given project. This file also specifies version constraints, ensuring that the correct versions of packages are installed. By managing dependencies in this way, developers can easily share and collaborate on projects without worrying about dependency mismatches.
Understanding Semantic Versioning
Semantic versioning (semver) is a system for versioning packages in a way that conveys compatibility information. Under this system, package versions consist of three parts: major, minor, and patch. A major version change indicates breaking changes, a minor version change indicates new features without breaking changes, and a patch version change indicates bug fixes. Understanding semver is crucial for managing dependencies and ensuring compatibility when upgrading packages.
Building a Simple Server with Node.js
One of the most common use cases for Node.js is building HTTP servers. Node.js makes this process simple and flexible, allowing developers to create servers tailored to their specific needs.
Creating a Basic HTTP Server
Creating a basic HTTP server in Node.js is straightforward. Using the ‘http’ module, developers can set up a server that listens on a specific port and handles incoming requests. This basic server can respond with static content or dynamically generate responses based on the incoming request. This flexibility allows developers to build everything from simple websites to complex APIs.
Using the ‘http’ Module
The ‘http’ module is central to building HTTP servers in Node.js. It provides methods for creating servers, handling requests, and sending responses. Developers can use this module to define custom routing logic, allowing the server to respond differently based on the requested URL. This module also supports HTTP methods like GET, POST, PUT, and DELETE, enabling the creation of RESTful endpoints.
Handling Routes and Responses
Handling routes in a Node.js server involves defining the logic for each URL path. Developers can use simple conditional statements to determine which response to send based on the incoming request. This approach allows for creating dynamic content and implementing complex routing logic. Responses can include static files, JSON data, or dynamically generated HTML.
Implementing RESTful Endpoints
RESTful endpoints are a common pattern in Node.js server development. These endpoints follow REST principles, allowing clients to interact with the server using standard HTTP methods. Implementing RESTful endpoints in Node.js involves defining specific routes for each resource and handling HTTP methods accordingly. This approach is ideal for building APIs that can be consumed by various clients, from web applications to mobile apps.
Working with Frameworks and Libraries
Node.js’s ecosystem is rich with frameworks and libraries that simplify development and enhance functionality. These tools can significantly accelerate the development process.
Popular Node.js Frameworks
Node.js frameworks provide a structured approach to building server-side applications. These frameworks often include features like routing, middleware, and templating, streamlining the development process. Popular frameworks include Express.js, Koa.js, and Hapi.js, each offering unique features and capabilities.
Overview of Express.js
Express.js is the most widely used Node.js framework, known for its simplicity and flexibility. It provides a minimalistic approach to building HTTP servers, allowing developers to define routes, middleware, and request handlers with ease. Express.js is highly extensible, with a vast collection of middleware available to add additional functionality.
Getting Started with Koa.js
Koa.js is another popular Node.js framework, created by the same team behind Express.js. It offers a more modern approach, focusing on modularity and async/await syntax. Koa.js allows developers to build lightweight, efficient servers with a focus on middleware composition. Its minimalist design provides a solid foundation for building custom server-side applications.
Other Useful Libraries for Node.js Development
Beyond frameworks, the Node.js ecosystem offers a wealth of libraries to enhance development. Popular libraries include ‘Lodash’ for utility functions, ‘Mongoose’ for MongoDB interactions, and ‘Socket.IO’ for real-time communication. These libraries streamline common tasks, allowing developers to focus on building unique functionality for their applications.
Testing and Debugging in Node.js
Testing and debugging are critical aspects of Node.js development. Robust testing ensures application reliability, while effective debugging helps identify and resolve issues.
Approaches to Testing
Node.js offers various approaches to testing, ranging from unit testing to integration testing. Unit testing involves testing individual components or functions to ensure they behave as expected. Integration testing involves testing the interaction between multiple components, ensuring that they work together correctly. Both approaches are essential for building reliable applications.
Unit Testing with Popular Libraries
Popular libraries for unit testing in Node.js include Mocha, Chai, and Jest. Mocha provides a flexible framework for defining and running unit tests, while Chai offers a rich set of assertion methods for verifying test outcomes. Jest, developed by Facebook, provides an all-in-one solution for unit testing with built-in support for mocking and code coverage.
Integration Testing Basics
Integration testing ensures that different parts of an application work together as intended. This approach is crucial for identifying issues that might not be apparent in unit tests. Tools like Supertest and Sinon help with integration testing, allowing developers to simulate HTTP requests and mock dependencies. Integration testing can be more complex than unit testing, but it’s essential for ensuring application stability.
Debugging Tools and Techniques
Debugging in Node.js involves identifying and resolving issues in the code. Node.js provides built-in debugging tools, allowing developers to set breakpoints, inspect variables, and step through code. Tools like ‘node-inspect’ and ‘Node.js Inspector’ offer powerful debugging capabilities, making it easier to identify and fix bugs. Additionally, developers can use logging frameworks like ‘Winston’ and ‘Bunyan’ to track application behavior and diagnose issues.
Deploying Node.js Applications
Deployment is the final stage of Node.js development, where the application is moved from development to production. Proper deployment ensures that the application runs smoothly and securely in a production environment.
Preparing for Deployment
Before deploying a Node.js application, developers must ensure it is production-ready. This preparation involves thorough testing, performance optimization, and proper configuration management. Additionally, developers should ensure that the application is scalable, allowing it to handle increased traffic and load.
Using Environment Variables
Environment variables are crucial for configuration management in production environments. By using environment variables, developers can separate configuration from code, making it easier to manage and secure. Environment variables are used for sensitive information like database credentials, API keys, and server configuration. This approach ensures that sensitive information is not hard-coded into the application.
Best Practices for Configuration
Proper configuration management is key to successful deployment. Best practices include using configuration files or environment variables, avoiding hard-coded credentials, and ensuring that sensitive information is encrypted. Additionally, developers should implement proper error handling and logging to track application behavior in production.
Securing Your Node.js Application
Security is paramount when deploying Node.js applications. Developers should follow best practices for securing server-side applications, including using secure connections (HTTPS), implementing authentication and authorization mechanisms, and protecting against common vulnerabilities like SQL injection and cross-site scripting (XSS). Regular security audits and code reviews can help identify and address security risks.
Conclusion
Node.js is a versatile and powerful platform for server-side development. Its event-driven architecture and non-blocking I/O model make it ideal for building scalable, high-performance applications. The Node.js ecosystem offers a wealth of frameworks, libraries, and tools, making it easy to create robust server-side applications. By following best practices for testing, debugging, and deployment, developers can build reliable and secure Node.js applications.
Key Takeaways from Node.js Fundamentals
Node.js offers unique advantages for server-side development, including its event-driven architecture and non-blocking I/O model. Understanding its architecture, modules, and asynchronous programming paradigms is crucial for leveraging its capabilities. Additionally, proper testing, debugging, and deployment practices are essential for building reliable applications.
Summary of Essential Concepts
Key concepts in Node.js include its event-driven architecture, asynchronous programming, and modularity. Understanding these concepts allows developers to build scalable and efficient applications. Additionally, using frameworks like Express.js and libraries like Mongoose and Socket.IO can streamline development and enhance functionality.