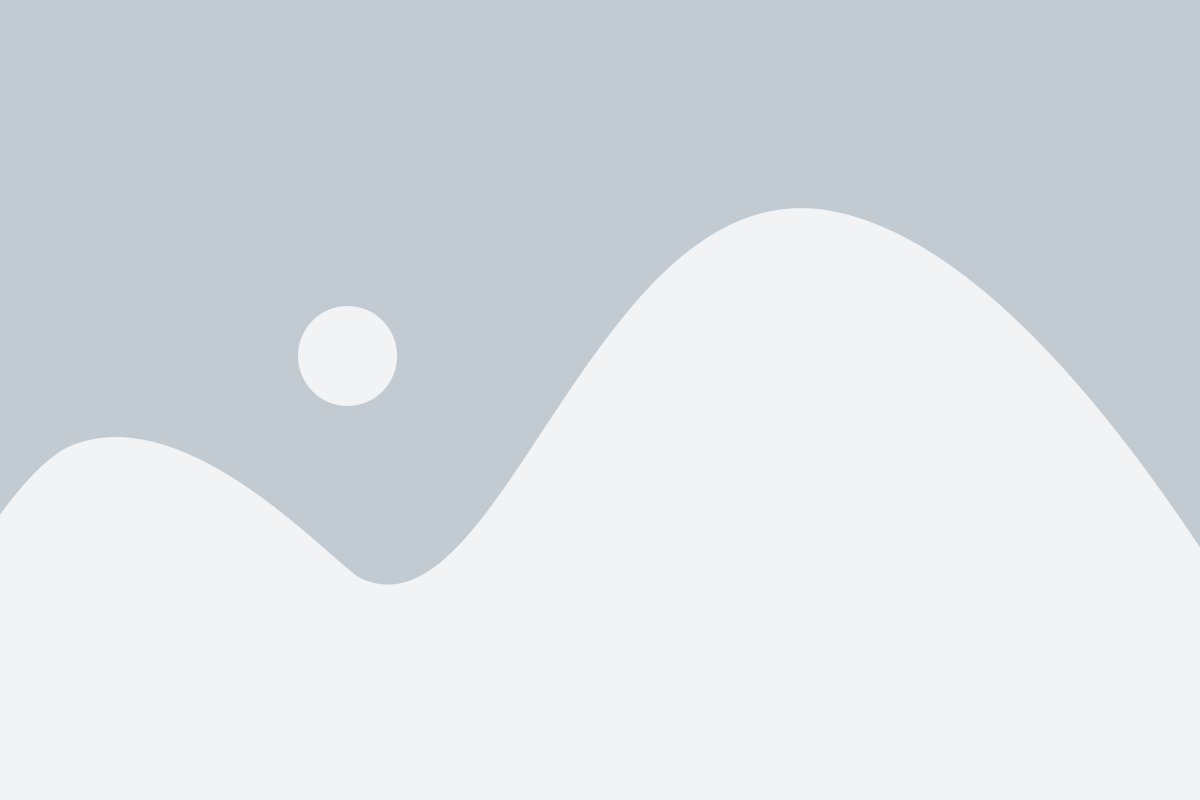
MongoDB is a popular NoSQL database that is widely used in modern full-stack applications. It pairs well with Python frameworks such as Django, Flask, and FastAPI. This guide will walk you through connecting MongoDB to a Python full-stack application.
- Setting Up MongoDB
Install MongoDB
For Linux (Ubuntu/Debian):
sudo apt update
sudo apt install -y mongodb
For macOS (using Homebrew):
brew tap mongodb/brew
brew install mongodb-community@6.0
For Windows, download and install MongoDB from the official website.
Start the MongoDB Service
sudo systemctl start mongod
Verify that MongoDB is running:
sudo systemctl status mongod
- Installing Required Python Packages
To interact with MongoDB in a Python full-stack application, install pymongo or mongoengine.
pip install pymongo
If using Django, install djongo for direct integration:
pip install djongo
- Configuring Python to Use MongoDB
Using PyMongo
Connect to MongoDB in Python:
from pymongo import MongoClient
client = MongoClient(“mongodb://localhost:27017/”)
db = client[“mydatabase”]
collection = db[“users”]
# Insert a document
collection.insert_one({“name”: “Alice”, “age”: 25})
# Retrieve documents
for user in collection.find():
print(user)
Using Django with Djongo
Modify settings.py:
DATABASES = {
‘default’: {
‘ENGINE’: ‘djongo’,
‘NAME’: ‘mydatabase’,
}
}
Run migrations (even though MongoDB is schema-less, Django requires it):
python manage.py makemigrations
python manage.py migrate
- Creating a Model and API with Flask
If using Flask, install Flask-PyMongo:
pip install flask flask-pymongo
Create a Flask API to interact with MongoDB:
from flask import Flask, jsonify, request
from flask_pymongo import PyMongo
app = Flask(__name__)
app.config[“MONGO_URI”] = “mongodb://localhost:27017/mydatabase”
mongo = PyMongo(app)
@app.route(“/users”, methods=[“GET”])
def get_users():
users = mongo.db.users.find()
return jsonify([{ “name”: user[“name”], “age”: user[“age”] } for user in users])
@app.route(“/users”, methods=[“POST”])
def add_user():
data = request.json
mongo.db.users.insert_one({“name”: data[“name”], “age”: data[“age”]})
return jsonify({“message”: “User added successfully!”})
if __name__ == “__main__”:
app.run(debug=True)
Run the Flask app:
python app.py
- Testing the API
Use curl or Postman to test the API:
curl -X POST -H “Content-Type: application/json” -d ‘{“name”: “Bob”, “age”: 30}’ http://127.0.0.1:5000/users
Conclusion
MongoDB is a powerful and flexible database for Python full-stack applications. Whether using PyMongo, Djongo with Django, or Flask-PyMongo, MongoDB enables efficient data storage and retrieval for modern web applications. 🚀