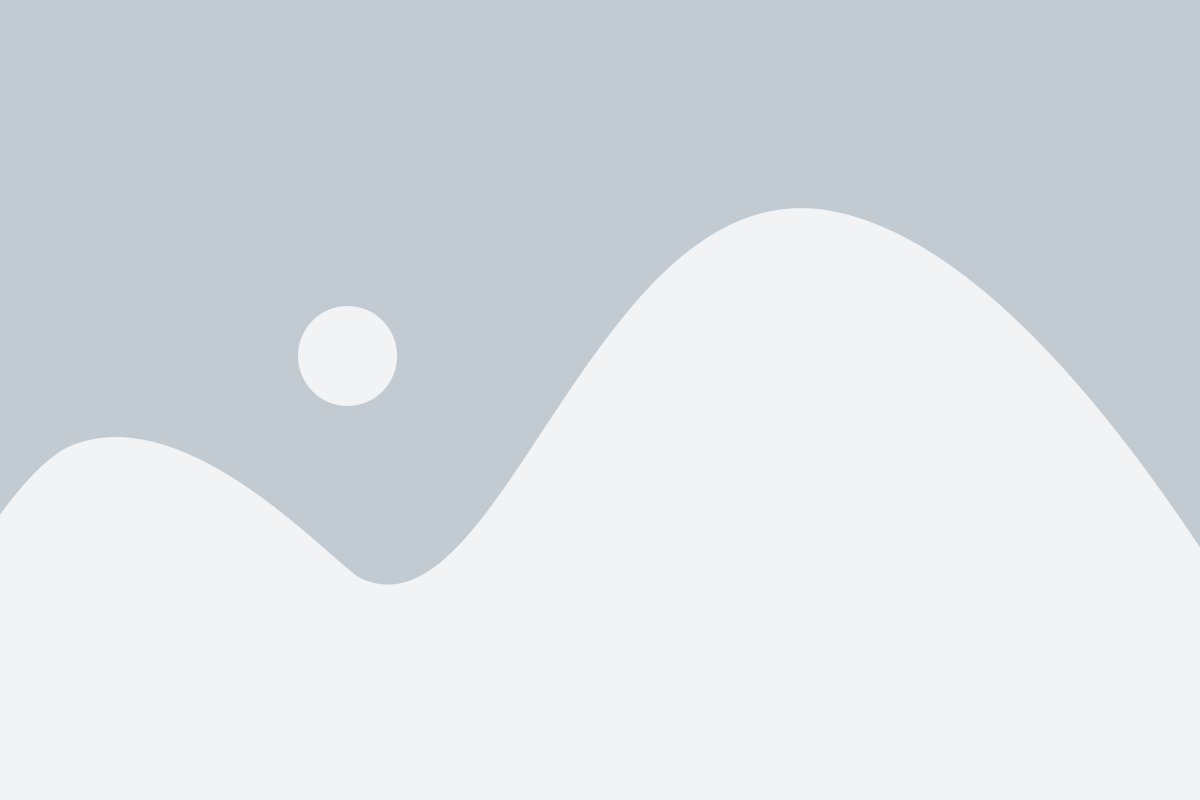
In today’s fast-paced world, automation is key to saving time and increasing efficiency. Python, with its simplicity and versatility, is the perfect language for automating everyday tasks. Whether you want to organize files, send emails, scrape data, or even control your smart devices, Python can handle it all. In this blog post, we’ll explore some essential Python scripts that can make your daily life easier.
- Automate File and Folder Organization
Manually sorting files can be tedious, especially if you deal with downloads, documents, and media files regularly. Python can help you organize files into relevant folders automatically.
Script to Organize Files by Type
python
import os
import shutil
source_folder = “C:/Users/YourName/Downloads” # Change this path
destination_folder = “C:/Users/YourName/Documents/Organized_Files”
file_types = {
“Images”: [“.jpg”, “.jpeg”, “.png”, “.gif”],
“Documents”: [“.pdf”, “.docx”, “.txt”],
“Videos”: [“.mp4”, “.mkv”, “.avi”],
}
for file in os.listdir(source_folder):
file_path = os.path.join(source_folder, file)
if os.path.isfile(file_path):
for category, extensions in file_types.items():
if file.endswith(tuple(extensions)):
folder_path = os.path.join(destination_folder, category)
os.makedirs(folder_path, exist_ok=True)
shutil.move(file_path, folder_path)
What It Does: Automatically sorts files into folders based on their extensions.
- Automate Sending Emails
Want to send an email reminder or daily report without manual effort? Python can automate emails using the smtplib library.
Script to Send Automated Emails
python
import smtplib
from email.mime.text import MIMEText
def send_email():
sender = “your_email@gmail.com”
receiver = “recipient_email@gmail.com”
subject = “Automated Email”
body = “Hello, this is an automated email sent using Python!”
msg = MIMEText(body)
msg[“From”] = sender
msg[“To”] = receiver
msg[“Subject”] = subject
with smtplib.SMTP_SSL(“smtp.gmail.com”, 465) as server:
server.login(sender, “your_password”) # Use an app password for security
server.sendmail(sender, receiver, msg.as_string())
send_email()
What It Does: Sends an email automatically without user intervention.
- Automate Web Scraping for Data Collection
Need to collect data from websites? Python’s BeautifulSoup and requests libraries make web scraping easy.
Script to Scrape Latest News Headlines
python
import requests
from bs4 import BeautifulSoup
url = “https://news.ycombinator.com/”
response = requests.get(url)
soup = BeautifulSoup(response.text, “html.parser”)
for i, item in enumerate(soup.find_all(“a”, class_=”storylink”), start=1):
print(f”{i}. {item.text} – {item[‘href’]}”)
What It Does: Fetches the latest news headlines from a website and prints them.
- Automate Screenshot Capture
If you frequently take screenshots, why not automate the process using Python?
Script to Take a Screenshot
python
import pyautogui
screenshot = pyautogui.screenshot()
screenshot.save(“screenshot.png”)
print(“Screenshot saved successfully!”)
What It Does: Captures a screenshot and saves it automatically.
- Automate PDF Merging
Handling multiple PDF files? Python can merge them into a single document.
Script to Merge PDFs
python from PyPDF2 import PdfMerger
pdfs = [“file1.pdf”, “file2.pdf”, “file3.pdf”]
merger = PdfMerger()
for pdf in pdfs:
merger.append(pdf)
merger.write(“merged_output.pdf”)
merger.close()
What It Does: Combines multiple PDFs into one file.
- Automate Social Media Posting
You can use Python to schedule and post content on Twitter or other social media platforms using APIs.
Script to Post a Tweet (Using Tweepy)
python
import tweepy
api_key = “your_api_key”
api_secret = “your_api_secret”
access_token = “your_access_token”
access_secret = “your_access_secret”
auth = tweepy.OAuth1UserHandler(api_key, api_secret, access_token, access_secret)
api = tweepy.API(auth)
api.update_status(“Hello, world! This is an automated tweet from Python.”)
What It Does: Automatically posts a tweet.
- Automate Reminders and Notifications
Want Python to remind you to take a break? You can set up desktop notifications.
Script to Show Desktop Notification
python from plyer import notification
notification.notify(
title=”Time to Take a Break!”,
message=”Stand up and stretch for a few minutes.”,
timeout=10
)
What It Does: Displays a notification on your desktop.
Conclusion
Vnet academy provide Python is a powerful tool for automating repetitive tasks, making life more efficient. Whether you need to organize files, send emails, scrape web data, or schedule reminders, Python has a solution for everything. By using these simple scripts, you can save time, boost productivity, and focus on more important tasks.