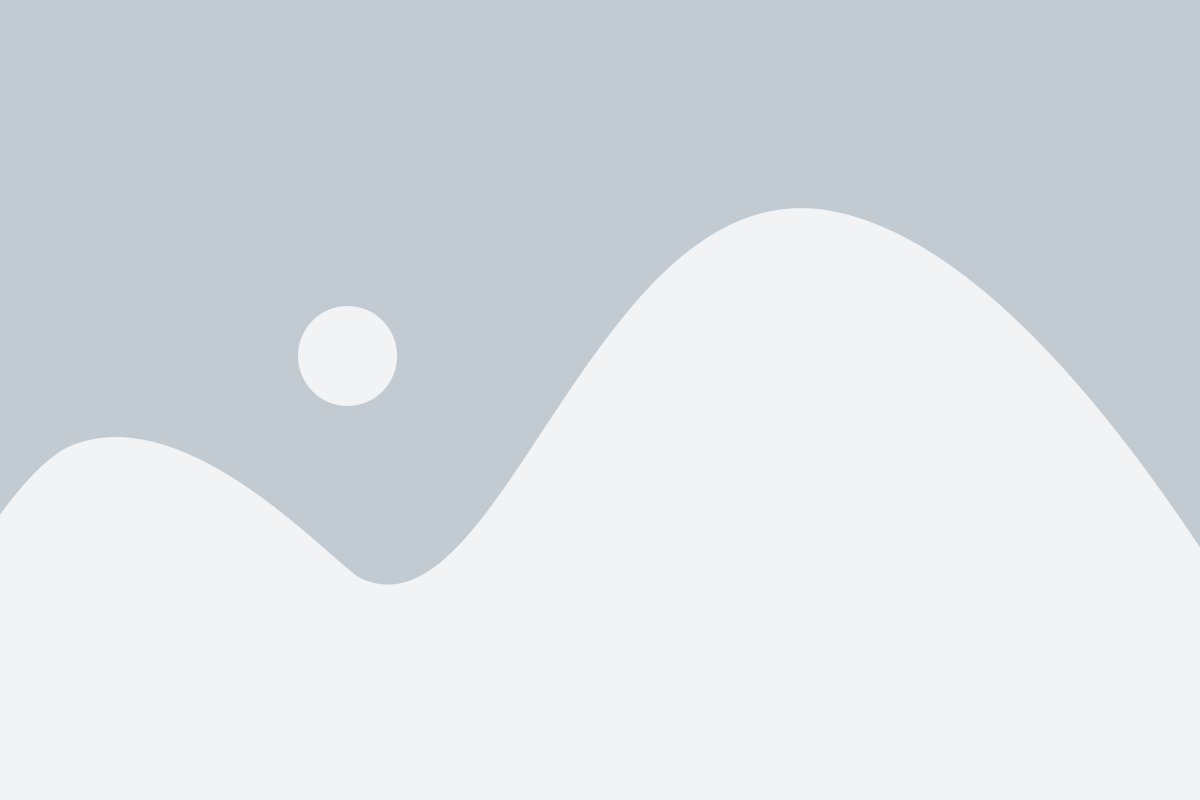
Why OpenCV is a Game-Changer for Image Processing In the realm of digital imaging, OpenCV stands as a technological marvel. Its ability to process, manipulate, and analyse images with remarkable precision has made it an indispensable tool for developers, researchers, and artists alike. Whether enhancing photos, detecting objects, or applying complex transformations, OpenCV empowers users to push the boundaries of visual creativity with ease.
Setting Up Your Python OpenCV Toolkit for Image Mastery Mastering OpenCV begins with a seamless setup. Ensuring a robust foundation will allow for smooth image processing and enable advanced manipulations without friction.
Installing OpenCV and Essential Libraries for Smooth Processing Begin by installing OpenCV via pip:
pip install opencv-python
Additional libraries such as NumPy, Matplotlib, and PIL (Pillow) provide enhanced capabilities, allowing for comprehensive image handling and visualization.
Understanding the Basics: Reading, Displaying, and Saving Images Reading an image is as simple as:
import cv2 image = cv2.imread(‘image.jpg’) cv2.imshow(‘Display Window’, image) cv2.waitKey(0) cv2.destroyAllWindows()
This fundamental operation opens the door to further modifications and enhancements.
Exploring Image Formats:
Choosing the Right One for Your Needs JPEG for compression, PNG for transparency, and TIFF for lossless quality—each format has its unique use case. Understanding when to use each ensures optimal results in various applications.
Transforming Images with Filters:
Add Depth, Texture, and Style Filters are powerful tools that alter image appearance, making them more visually striking or optimized for analysis.
Sharpen and Blur:
Enhance or Soften Images with Precision Using Gaussian blur or sharpening filters can make images more dynamic or soften harsh details for a polished effect.
blurred = cv2.GaussianBlur(image, (5,5), 0) sharpen_kernel = np.array([[0, -1, 0], [-1, 5, -1], [0, -1, 0]]) sharpened = cv2.filter2D(image, -1, sharpen_kernel)
Colour Manipulation:
Adjust Hue, Saturation, and Brightness Like a Pro Adjusting color properties can dramatically change the visual impact of an image. OpenCV’s HSV model allows for fine-tuned adjustments.
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV) hsv[…,2] = hsv[…,2] * 1.2 # Increasing brightness image = cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)
Edge Detection Mastery:
Highlight Key Features with Sobel and Canny Detecting edges enhances image clarity for feature extraction.
edges = cv2.Canny(image, 100, 200)
Seamless Edits:
Modify, Crop, and Resize with Precision Transformations like cropping, resizing, and rotating is essential for refining images.
Cropping Magic: Focus on What Matters in an Image Cropping allows you to highlight specific areas:
cropped = image[50:200, 100:300]
Resizing Techniques:
Maintain Quality While Adjusting Dimensions Maintaining aspect ratios ensures images remain visually appealing.
resized = cv2.resize(image, (width, height), interpolation=cv2.INTER_LINEAR)
Image Rotation and Flipping: Create Stunning Perspectives
rotated = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE) flipped = cv2.flip(image, 1) # Horizontal Flip
Stunning Effects:
Elevate Images with Next-Level Enhancements Advanced techniques allow for dramatic image transformations.
Artistic Transformations: Turn Photos into Sketches, Cartoons, and More Convert images into stunning effects:
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) sketch = cv2.Canny(gray, 50, 150)
Background Removal:
Extract Objects with Smart Masking Techniques Using OpenCV’s contour detection, background removal becomes effortless.
Adding Text and Shapes: Overlay Graphics for Professional Results
cv2.putText(image, ‘OpenCV Magic’, (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 0, 0), 2)
Advanced OpenCV Techniques:
AI-Powered Enhancements Modern AI-driven capabilities elevate OpenCV’s potential.
Noise Reduction and Image Denoising for Crystal-Clear Output Techniques like Non-Local Means Denoising refine image quality.
Object Detection and Face Recognition for Smart Processing Integrating deep learning models enables facial and object recognition.
Image Stitching:
Create Panoramas with Seamless Blending Multiple images can be stitched together to create breathtaking panoramas.
Real-World Applications of Python OpenCV Image Transformations Practical applications make OpenCV an invaluable tool.
Enhancing Social Media Images for Maximum Engagement Filters, overlays, and optimizations make images more shareable.
Automating Image Processing for Web and Mobile Applications Automated resizing and compression improve performance and UX.
Leveraging OpenCV in AI and Machine Learning Projects Feature extraction and classification unlock powerful AI applications.
Optimizing Performance:
Speed Up Image Processing Like a Pro Efficiency is key in large-scale image processing tasks.
Using Multi-Threading for Faster Processing Parallel processing optimizes performance for bulk image handling.
Leveraging GPU Acceleration for High-Resolution Images CUDA-enabled OpenCV operations significantly improve execution speed.
Memory Management Tricks for Efficient Computation Optimizing array operations reduces unnecessary memory consumption.
Master Python OpenCV:
Next Steps to Take Your Skills Further Push your OpenCV expertise to the next level.
Exploring OpenCV with Deep Learning for Image Recognition Integrate OpenCV with TensorFlow or PyTorch for state-of-the-art results.
Experimenting with Custom Filters for Unique Image Styles Creating personalized filters adds a distinctive touch to images.
Joining the OpenCV Community and Contributing to Open-Source Projects Engaging with the OpenCV community fosters learning and innovation.
Conclusion:
Python OpenCV is an incredibly powerful tool for image processing, offering a vast range of functionalities from basic transformations to advanced AI-powered enhancements. Whether you are a beginner looking to perform simple edits or a professional diving into deep learning integrations, OpenCV provides the flexibility and efficiency needed to achieve stunning results. By continuously exploring its capabilities and optimizing performance, you can elevate your image processing skills and bring your creative visions to life.