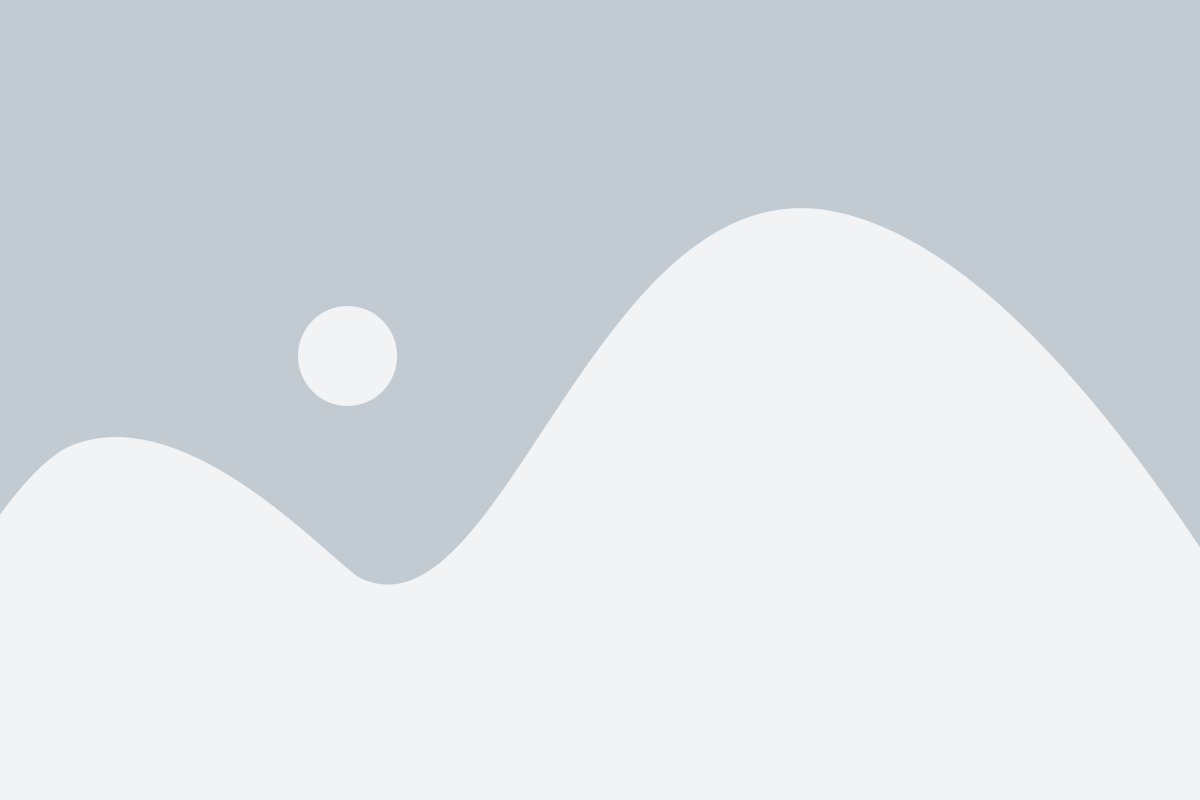
Object tracking is a fundamental concept in computer vision that enables applications like surveillance, robotics, and augmented reality. With OpenCV, you can easily implement real-time object tracking using various tracking algorithms.
Step 1: Install OpenCV
Ensure you have OpenCV installed. If not, install it using:
pip install opencv-python opencv-contrib-python
Step 2: Load the Video Stream
To track objects in real-time, we use a webcam feed or a video file.
import cv2
# Open webcam
cap = cv2.VideoCapture(0)
if not cap.isOpened():
print(“Error: Could not open webcam.”)
exit()
Step 3: Select Object to Track
Manually select an object in the first frame.
# Read the first frame
ret, frame = cap.read()
if not ret:
print(“Error: Could not read frame.”)
cap.release()
exit()
# Select region of interest (ROI) for tracking
bbox = cv2.selectROI(“Select Object”, frame, False)
cv2.destroyWindow(“Select Object”)
Step 4: Initialize Object Tracker
OpenCV provides different tracking algorithms, such as MIL, KCF, and CSRT.
# Create a tracker (you can choose different algorithms)
tracker = cv2.TrackerCSRT_create()
tracker.init(frame, bbox)
Step 5: Perform Real-Time Tracking
We continuously update the tracker and display results.
while True:
ret, frame = cap.read()
if not ret:
break
# Update tracker
success, bbox = tracker.update(frame)
if success:
x, y, w, h = map(int, bbox)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
else:
cv2.putText(frame, “Tracking lost”, (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 0, 255), 2)
cv2.imshow(“Object Tracking”, frame)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
Conclusion
With just a few lines of code, you can implement real-time object tracking using OpenCV. Experiment with different trackers like MIL, KCF, and CSRT to see which works best for your application. Happy coding!