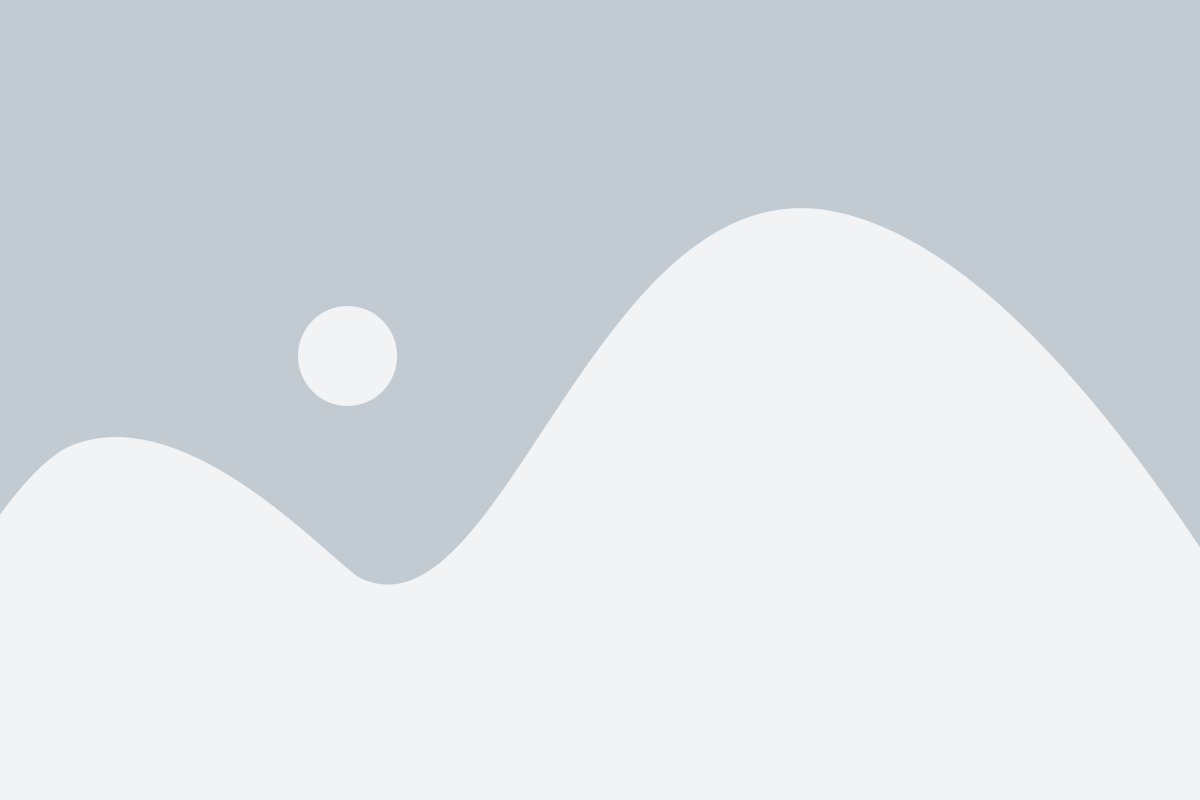
Motion detection is a fundamental aspect of computer vision applications such as surveillance, security systems, and automated monitoring. Using OpenCV, we can implement a simple motion detection system that identifies changes in a video stream.
Step 1: Install OpenCV
Ensure you have OpenCV installed before proceeding. If not, install it using:
pip install opencv-python
Step 2: Capture Video Stream
We will start by capturing the video stream from a webcam or a pre-recorded video.
import cv2
# Capture video from the webcam
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
cv2.imshow(“Video Stream”, frame)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
Step 3: Convert Frames to Grayscale and Apply Gaussian Blur
To reduce noise and improve motion detection accuracy, we convert frames to grayscale and apply Gaussian blur.
def preprocess_frame(frame):
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
gray = cv2.GaussianBlur(gray, (21, 21), 0)
return gray
Step 4: Detect Motion
We compare the current frame with the previous frame to detect changes.
first_frame = None
while True:
ret, frame = cap.read()
if not ret:
break
gray = preprocess_frame(frame)
if first_frame is None:
first_frame = gray
continue
frame_diff = cv2.absdiff(first_frame, gray)
thresh = cv2.threshold(frame_diff, 25, 255, cv2.THRESH_BINARY)[1]
thresh = cv2.dilate(thresh, None, iterations=2)
cv2.imshow(“Motion Detection”, thresh)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
Step 5: Highlight Motion Using Contours
We use contours to highlight areas where motion is detected.
import numpy as np
while True:
ret, frame = cap.read()
if not ret:
break
gray = preprocess_frame(frame)
if first_frame is None:
first_frame = gray
continue
frame_diff = cv2.absdiff(first_frame, gray)
thresh = cv2.threshold(frame_diff, 25, 255, cv2.THRESH_BINARY)[1]
thresh = cv2.dilate(thresh, None, iterations=2)
contours, _ = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
if cv2.contourArea(contour) < 500:
continue
(x, y, w, h) = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imshow(“Motion Detection”, frame)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
Conclusion
With OpenCV, you can easily implement a real-time motion detection system by processing video frames, detecting changes, and highlighting motion regions using contours. You can further enhance this system by integrating it with alarms, notifications, or object tracking. Try experimenting with different threshold values and blur settings to refine detection accuracy!