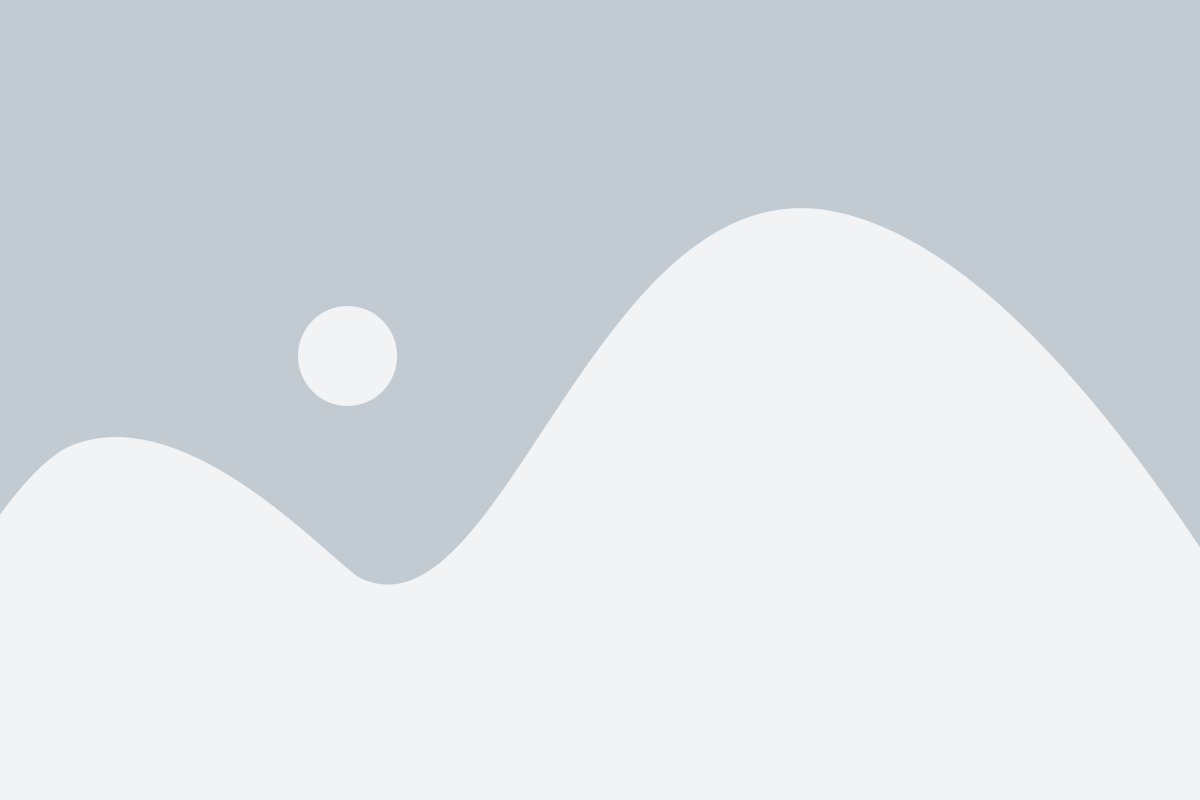
Python’s OpenCV library is a powerful tool for image processing, offering a wide range of functions to manipulate and transform images effortlessly. Whether you’re a beginner or an experienced developer, OpenCV allows you to apply effects, enhance images, and extract useful information with just a few lines of code. In this article, we’ll explore some of the most useful OpenCV techniques that can transform your images like a pro.
- Reading and Displaying Images
Before applying any transformations, we first need to load and display images using OpenCV.
Code Example:
import cv2
image = cv2.imread(‘image.jpg’)
cv2.imshow(‘Original Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Converting to Grayscale
Many image processing tasks require grayscale images. Converting an image to grayscale reduces computational complexity and enhances edge detection.
Code Example:
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow(‘Grayscale Image’, gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Resizing and Cropping
Resizing and cropping images are essential for pre-processing before feeding them into a model.
Code Example:
resized = cv2.resize(image, (300, 300))
cropped = image[50:200, 100:300]
cv2.imshow(‘Resized Image’, resized)
cv2.imshow(‘Cropped Image’, cropped)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Applying Filters (Blurring and Sharpening)
Blurring smooths out noise, while sharpening enhances edges.
Blurring Example:
blurred = cv2.GaussianBlur(image, (15, 15), 0)
cv2.imshow(‘Blurred Image’, blurred)
cv2.waitKey(0)
cv2.destroyAllWindows()
Sharpening Example:
import numpy as np
kernel = np.array([[0, -1, 0], [-1, 5,-1], [0, -1, 0]])
sharpened = cv2.filter2D(image, -1, kernel)
cv2.imshow(‘Sharpened Image’, sharpened)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Edge Detection with Canny Algorithm
Edge detection is useful for object detection and feature extraction.
Code Example:
edges = cv2.Canny(image, 100, 200)
cv2.imshow(‘Edges’, edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Image Thresholding for Binarization
Thresholding converts images into binary format, which is useful for shape detection.
Code Example:
_, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
cv2.imshow(‘Binary Image’, binary)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Contour Detection
Contours are useful for detecting objects in an image.
Code Example:
contours, _ = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(image, contours, -1, (0, 255, 0), 2)
cv2.imshow(‘Contours’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Morphological Transformations (Erosion & Dilation)
Erosion and dilation are used to enhance or suppress image features.
Erosion Example:
kernel = np.ones((5,5), np.uint8)
eroded = cv2.erode(binary, kernel, iterations=1)
cv2.imshow(‘Eroded Image’, eroded)
cv2.waitKey(0)
cv2.destroyAllWindows()
Dilation Example:
dilated = cv2.dilate(binary, kernel, iterations=1)
cv2.imshow(‘Dilated Image’, dilated)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Image Perspective Transformation
Perspective transformation allows us to change the viewpoint of an image.
Code Example:
pts1 = np.float32([[50, 50], [200, 50], [50, 200], [200, 200]])
pts2 = np.float32([[10, 100], [180, 50], [100, 250], [250, 250]])
M = cv2.getPerspectiveTransform(pts1, pts2)
warped = cv2.warpPerspective(image, M, (300, 300))
cv2.imshow(‘Warped Image’, warped)
cv2.waitKey(0)
cv2.destroyAllWindows()
- Face Detection with OpenCV
OpenCV has a built-in face detector that can be used to detect faces in an image.
Code Example:
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + ‘haarcascade_frontalface_default.xml’)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow(‘Face Detection’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Conclusion
With OpenCV, you can perform a wide range of image transformations to enhance, analyze, and manipulate images efficiently. Whether you’re working on a computer vision project or just exploring image processing, OpenCV provides a simple yet powerful framework to get started. Experiment with these techniques and take your image processing skills to the next level!