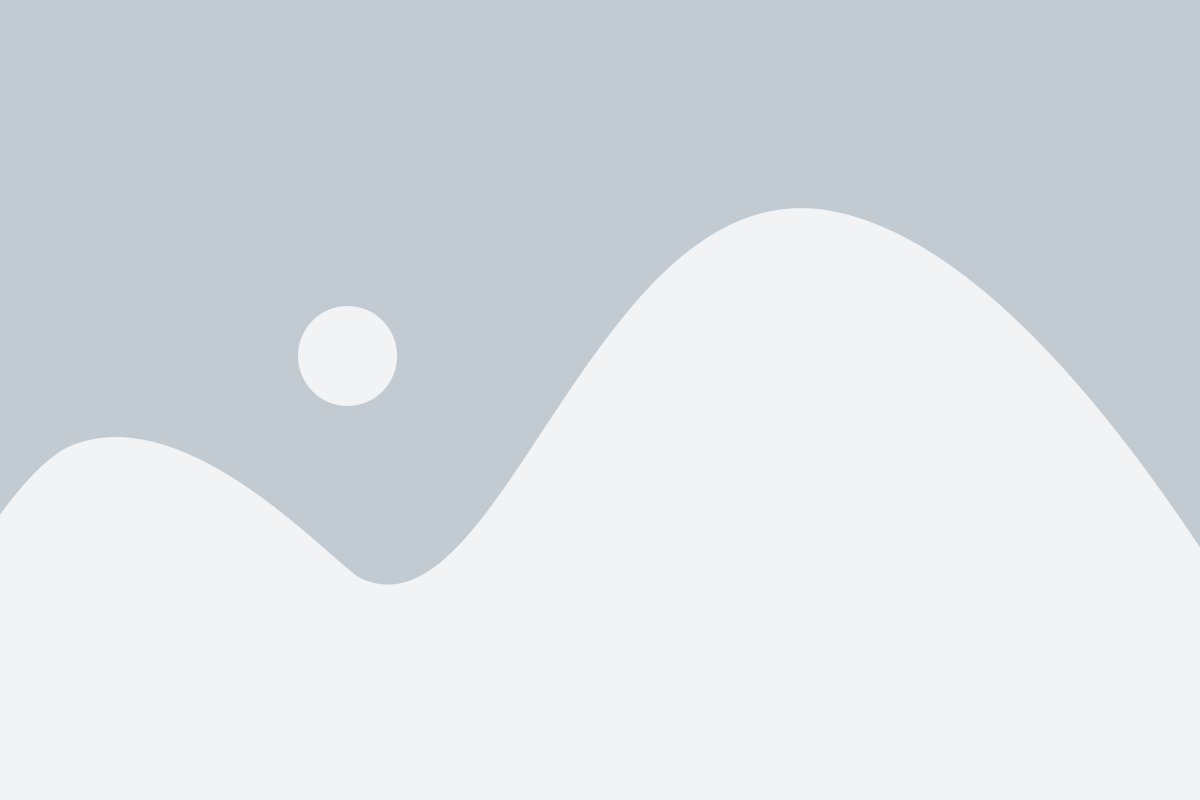
Edge detection is a crucial technique in computer vision, widely used in applications like object detection, image segmentation, and feature extraction. OpenCV makes it simple to implement edge detection with powerful algorithms like the Canny Edge Detector.
Step 1: Install OpenCV
Before we begin, ensure you have OpenCV installed. If not, install it using:
pip install opencv-python
Step 2: Load and Convert Image to Grayscale
Since edge detection works best in grayscale, we first load the image and convert it.
import cv2
# Load the image
image = cv2.imread(‘image.jpg’)
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Step 3: Apply Gaussian Blur
Blurring helps to reduce noise and improve edge detection accuracy.
# Apply Gaussian blur
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
Step 4: Perform Edge Detection Using Canny
The Canny edge detector is one of the most widely used edge detection techniques.
# Apply Canny Edge Detection
edges = cv2.Canny(blurred, 50, 150)
Step 5: Display the Result
cv2.imshow(‘Edges’, edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
Bonus: Edge Detection in Real-Time (Webcam)
To detect edges in real-time using a webcam, use the following code:
# Open webcam
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
edges = cv2.Canny(blurred, 50, 150)
cv2.imshow(‘Real-Time Edge Detection’, edges)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
Conclusion
In just a few steps, you’ve mastered edge detection using OpenCV. This technique is essential for various image processing applications, from object recognition to medical imaging. Experiment with different threshold values to fine-tune detection for different images!