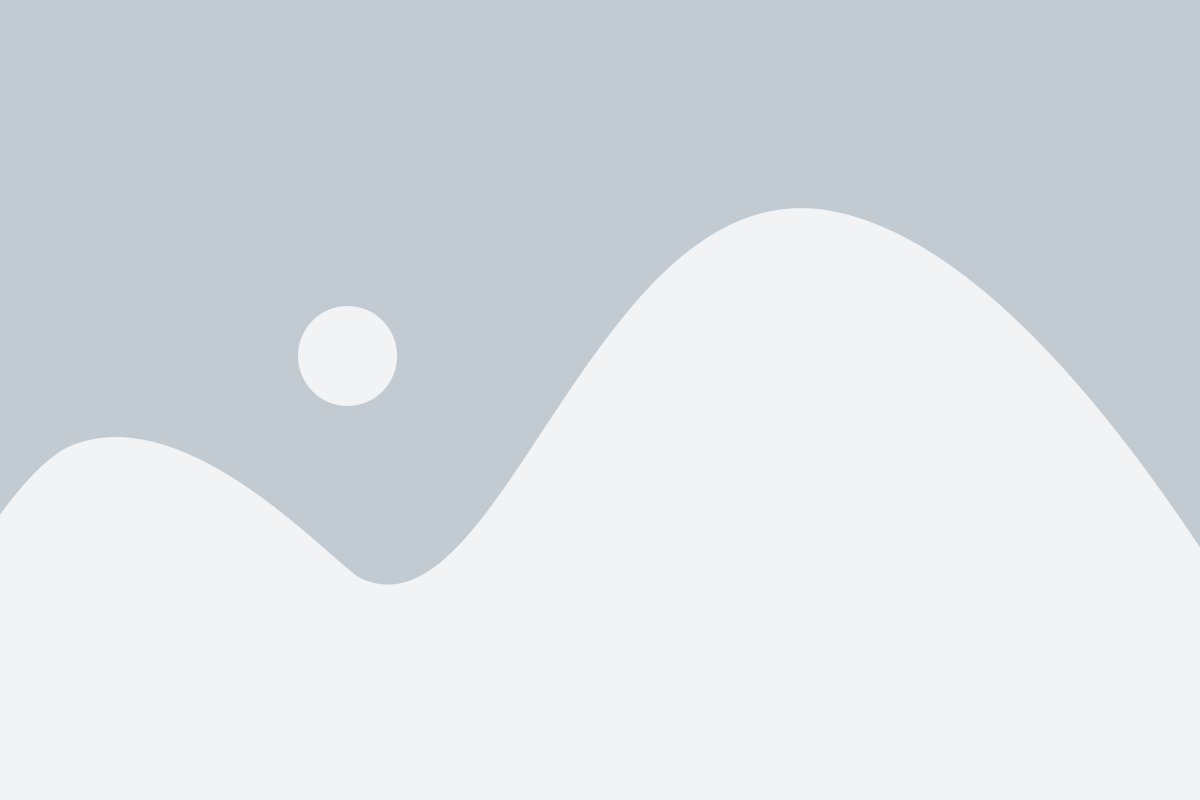
Python has become one of the most popular programming languages for web development due to its simplicity, readability, and vast ecosystem of frameworks and libraries. Whether you are a beginner looking to build your first website or an aspiring developer exploring web technologies, Python provides a solid foundation for web development. In this guide, we’ll explore the basics of Python web development, popular frameworks, and how to get started.
Why Choose Python for Web Development?
Python is widely used for web development because of:
✅ Ease of Learning – Python’s clean and readable syntax makes it beginner-friendly.
✅ Extensive Frameworks – Frameworks like Django and Flask simplify web development.
✅ Scalability – Python supports both small projects and large-scale applications.
✅ Strong Community Support – A vast community of developers ensures continuous updates and improvements.
✅ Versatile Applications – From simple websites to complex web applications, Python can handle it all.
Understanding Web Development with Python
Web development consists of two main parts:
- Frontend Development – Involves designing the user interface using HTML, CSS, and JavaScript.
- Backend Development – Handles server-side logic, database interactions, and API communication using Python.
Python is mainly used for backend development, where it processes user requests, manages data, and interacts with databases.
Choosing the Right Python Web Framework
Python offers several frameworks for web development, but the most popular ones are:
- Django – The Complete Web Framework
Django is a high-level web framework that follows the “batteries-included” philosophy, providing built-in tools for database management, authentication, and security.
Why Choose Django?
✔ Fast development with built-in features
✔ Secure with built-in protection against attacks
✔ Scalable for handling large projects
Installation:
bash
pip install django
Basic Django Project Setup:
bash
django-admin startproject mywebsite
cd mywebsite
python manage.py runserver
- Flask – The Lightweight Microframework
Flask is a minimalistic framework that provides the essential tools needed for web development without unnecessary complexity.
Why Choose Flask?
✔ Lightweight and flexible
✔ Easy to learn and great for small projects
✔ Allows full customization
Installation:
bash
pip install flask
Basic Flask Web App:
python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == “__main__”:
app.run(debug=True)
Run the script, and visit http://127.0.0.1:5000/ in your browser to see the output.
Working with Databases in Python Web Development
Web applications often require databases to store and manage data. Python supports multiple databases, including:
- SQLite (built-in and lightweight)
- PostgreSQL (powerful and scalable)
- MySQL (widely used for web applications)
Using Django with a Database
Django comes with a built-in ORM (Object-Relational Mapper) to interact with databases easily.
python
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=255)
content = models.TextField()
published_date = models.DateTimeField(auto_now_add=True)
Run migrations to create the database tables:
bash
python manage.py makemigrations
python manage.py migrate
Using Flask with a Database (SQLAlchemy)
Flask doesn’t come with a built-in ORM, but you can use SQLAlchemy to manage database interactions.
Installation:
bash
pip install flask-sqlalchemy
Example Database Model in Flask:
python
from flask_sqlalchemy import SQLAlchemy
app.config[‘SQLALCHEMY_DATABASE_URI’] = ‘sqlite:///site.db’
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(100), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
Building APIs with Python
APIs (Application Programming Interfaces) allow communication between the frontend and backend. Python makes API development easy using Flask and Django REST Framework (DRF).
Creating an API with Flask
python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route(‘/api’, methods=[‘GET’])
def api():
return jsonify({“message”: “Hello, API!”})
if __name__ == “__main__”:
app.run(debug=True)
Run the script and visit http://127.0.0.1:5000/api to see the JSON response.
Creating an API with Django REST Framework
bash
pip install djangorestframework
Basic Django API View:
python
from rest_framework.response import Response
from rest_framework.decorators import api_view
@api_view([‘GET’])
def api_home(request):
return Response({“message”: “Hello, API!”})
Deploying a Python Web Application
Once your web app is ready, you can deploy it online using:
- Heroku – Easy deployment for Flask/Django apps
- AWS – Scalable cloud hosting
- DigitalOcean – Affordable cloud hosting
- PythonAnywhere – Great for beginners
Deploying a Flask App on Heroku
- Install Heroku CLI and login:
bash
heroku login
- Create a requirements.txt file:
bash
pip freeze > requirements.txt
- Create a Procfile:
makefile
web: gunicorn app:app
- Deploy the app:
bash
git init
git add .
git commit -m “Initial commit”
heroku create my-flask-app
git push heroku master
heroku open
Conclusion
Python is a fantastic choice for web development, offering powerful frameworks like Django and Flask to build dynamic web applications. Whether you’re developing a simple blog or a complex e-commerce site, Python’s versatility and ease of use make it an excellent choice for beginners and professionals alike.