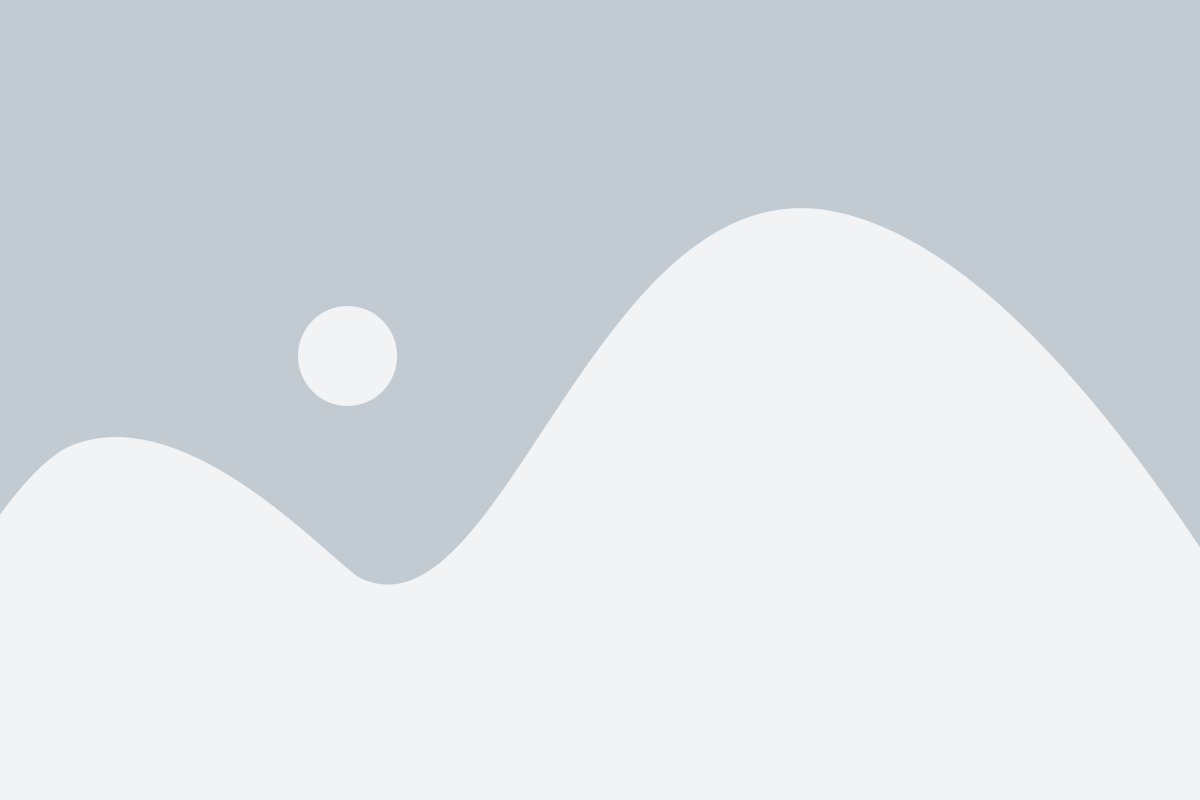
Introducing Node.js as a game-changer in backend development
Node.js has emerged as a transformative force in backend development, revolutionizing the way developers approach building scalable and efficient server-side applications. Its innovative architecture and powerful features have propelled it to the forefront of the development landscape, offering a modern solution to the challenges of backend programming.
Why Node.js is gaining popularity among developers
Node.js is rapidly gaining popularity among developers due to its unique features and advantages. Its asynchronous, event-driven architecture allows for non-blocking I/O operations, making it highly efficient and scalable. Additionally, its use of JavaScript as the primary language enables developers to build both frontend and backend components with a single language, streamlining the development process and promoting code reusability.
Understanding Node.js
What is Node.js and its role in backend development?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a web browser. It is commonly used for building server-side applications and APIs, handling tasks such as file I/O, networking, and database operations. Its lightweight, event-driven architecture makes it well-suited for building real-time applications and handling concurrent connections efficiently.
Exploring the advantages of using Node.js for backend development
Node.js offers several advantages for backend development, including its asynchronous, non-blocking nature, which allows for handling multiple requests simultaneously without blocking the execution thread. This enables the creation of highly responsive and scalable applications. Additionally, Node.js benefits from a vibrant ecosystem of libraries and frameworks, such as Express.js, which further accelerates development and enhances functionality.
Getting Started with Node.js
Setting up Node.js development environment
Setting up a Node.js development environment is straightforward and involves installing Node.js and a code editor. Node.js can be downloaded from the official website or installed via package managers like npm or yarn. Once installed, developers can use npm to manage project dependencies and packages, enhancing productivity and collaboration.
Installing Node.js on different operating systems
Node.js is compatible with major operating systems, including Windows, macOS, and Linux. Installation instructions may vary slightly depending on the operating system, but generally involve downloading the appropriate installer from the Node.js website and following the on-screen instructions. Once installed, developers can start writing and executing JavaScript code using Node.js’ runtime environment.
Configuring tools and dependencies for Node.js development
Configuring tools and dependencies for Node.js development is essential for a smooth development workflow. Developers can use npm to install project dependencies and manage packages, enabling them to leverage third-party libraries and frameworks to enhance application functionality. Additionally, code editors like Visual Studio Code or Atom provide features such as syntax highlighting, code completion, and debugging tools, further improving productivity and code quality.
Asynchronous Programming in Node.js
Understanding the asynchronous, non-blocking nature of Node.js
Node.js’ asynchronous, non-blocking nature is a core feature that sets it apart from traditional server-side technologies. Instead of waiting for I/O operations to complete before moving on to the next task, Node.js continues executing code while handling I/O operations in the background. This enables it to handle multiple requests simultaneously without blocking the execution thread, resulting in improved performance and scalability.
Exploring callbacks, promises, and async/await for handling asynchronous operations
Node.js offers several mechanisms for handling asynchronous operations, including callbacks, promises, and async/await. Callbacks are a traditional approach where a function is passed as an argument to another function to be executed later. Promises provide a more elegant solution for managing asynchronous operations by representing a value that may be available in the future. async/await is a syntactic sugar that simplifies working with promises, making asynchronous code more readable and maintainable.
Best practices for writing asynchronous code in Node.js
When writing asynchronous code in Node.js, it’s essential to follow best practices to ensure code readability, maintainability, and performance. This includes properly handling errors using try/catch blocks or error-first callbacks, avoiding callback hell by using named functions or promises, and optimizing performance by minimizing blocking operations and leveraging asynchronous APIs whenever possible.
Working with Modules in Node.js
Understanding the module system in Node.js
Node.js’ module system allows developers to organize code into reusable modules, promoting code reusability and maintainability. Modules encapsulate related functionality and expose a public interface for other modules to consume. By breaking down applications into smaller, modular components, developers can write cleaner, more maintainable code and promote code reuse across projects.
Creating and exporting modules for code organization and reusability
Creating and exporting modules in Node.js is a straightforward process. Developers can define a module by creating a JavaScript file and using the module.exports or exports object to expose functions, objects, or variables for use by other modules. By encapsulating related functionality within modules, developers can create modular applications that are easier to understand, maintain, and extend.
Leveraging built-in and third-party modules to enhance backend functionality
In addition to built-in modules provided by Node.js, developers can leverage a vast ecosystem of third-party modules available via npm to enhance the functionality and productivity of their applications. npm, the Node.js package manager, provides access to thousands of libraries and tools for various use cases, ranging from web development frameworks like Express.js to utility libraries like Lodash. By leveraging third-party modules, developers can accelerate development, reduce development time, and focus on building core application logic.
Mastering Core Modules in Node.js
Exploring core modules such as fs, http, and path for file I/O, networking, and path manipulation
Node.js provides a set of core modules that offer essential functionality for common tasks such as file I/O, networking, and path manipulation. Some of the core modules provided by Node.js include fs (file system), http (HTTP server), https (HTTPS server), and path (path manipulation). These core modules are built into Node.js and can be used directly in applications without the need for external dependencies.
Understanding the functionalities and best practices for utilizing core modules in Node.js applications
Each core module in Node.js offers a range of functionalities and methods for performing specific tasks. For example, the fs module provides methods for reading and writing files, creating directories, and manipulating file attributes. The http module allows developers to create HTTP servers and handle incoming requests, while the path module provides utilities for working with file and directory paths. By understanding the functionalities of core modules, developers can leverage them effectively to build robust and efficient applications.
Building RESTful APIs with Express.js
Introduction to Express.js as a minimalist web framework for Node.js
Express.js is a minimalist web framework for Node.js that simplifies the process of building RESTful APIs. It provides a lightweight and flexible architecture for defining routes, handling requests, and managing middleware. With its streamlined approach to web development, Express.js allows developers to focus on building scalable and efficient APIs without unnecessary boilerplate code.
Designing RESTful APIs with Express.js: routes, middleware, and request handling
Designing RESTful APIs with Express.js involves defining routes for handling HTTP requests, implementing middleware for request processing, and managing request and response objects. Routes are defined using HTTP methods such as GET, POST, PUT, and DELETE, and are associated with specific URL paths. Middleware functions can be used to perform tasks such as request validation, authentication, and error handling, while route handlers process incoming requests and generate appropriate responses.
Testing and debugging Express.js APIs for reliability and performance
Testing and debugging Express.js APIs is essential for ensuring reliability and performance. Unit tests can be written using testing frameworks like Mocha or Jest to validate individual components and functions, while integration tests can be used to verify the behavior of the API as a whole. Additionally, debugging tools such as Chrome DevTools or VS Code debugger can be used to inspect and troubleshoot code during development.
Integrating Databases with Node.js
Integrating Node.js with databases like MongoDB, MySQL, and PostgreSQL
Node.js provides support for integrating with various databases, including MongoDB, MySQL, and PostgreSQL, allowing developers to build data-driven applications with ease. By leveraging database drivers and ORMs (Object-Relational Mapping), developers can interact with databases, perform CRUD operations, and execute complex queries from Node.js applications. This section explores different approaches to integrating databases with Node.js, best practices for database interactions, and tips for optimizing database performance and scalability.
Working with database drivers and ORMs for CRUD operations and data querying
Database drivers and ORMs (Object-Relational Mapping) provide convenient abstractions for interacting with databases from Node.js applications. Drivers allow developers to execute SQL queries directly against the database, while ORMs provide higher-level abstractions that map database tables to JavaScript objects. By using database drivers and ORMs, developers can simplify database interactions, reduce boilerplate code, and focus on building application logic.
Best practices for database interactions and optimizing database performance
When working with databases in Node.js applications, it’s important to follow best practices to ensure reliability, performance, and security. This includes properly handling database connections, using parameterized queries to prevent SQL injection attacks, and implementing indexes and query optimizations to improve performance. Additionally, developers should consider factors such as data modeling, transaction management, and error handling to build robust and efficient database-driven applications.
Deploying Node.js Applications
Preparing Node.js applications for deployment to production environments
Preparing Node.js applications for deployment involves optimizing performance, configuring environment variables, and securing sensitive information. This includes minimizing dependencies, bundling and minifying assets, and setting up error logging and monitoring. Additionally, developers should consider factors such as scalability, availability, and disaster recovery to ensure that applications perform reliably in production environments.
Choosing the right hosting platform for Node.js applications
Choosing the right hosting platform is crucial for deploying Node.js applications effectively. Options range from traditional virtual private servers (VPS) to managed platforms like Heroku or AWS Elastic Beanstalk. Factors to consider include scalability, pricing, deployment options, and support for Node.js features and dependencies. By selecting the appropriate hosting platform, developers can ensure that their applications are stable, secure, and performant in production environments.
Configuring continuous deployment pipelines for automated deployments
Configuring continuous deployment pipelines automates the process of deploying Node.js applications, reducing the risk of human error and streamlining the release process. Tools like Jenkins, Travis CI, or GitHub Actions can be used to set up automated build, test, and deployment pipelines that trigger on code changes. By integrating continuous deployment into the development workflow, developers can accelerate the release cycle and ensure that applications are deployed consistently and reliably.
Conclusion
Recap of key concepts and benefits of using Node.js for backend development
In conclusion, Node.js offers a powerful and flexible platform for building scalable and efficient backend applications. Its asynchronous, event-driven architecture enables developers to handle concurrent connections and I/O operations efficiently, resulting in highly responsive and performant applications. By leveraging its rich ecosystem of modules, frameworks, and tools, developers can streamline development workflows, enhance productivity, and build innovative solutions to meet the demands of modern web development.
Encouraging further exploration and learning opportunities in Node.js
As the Node.js ecosystem continues to evolve, there are endless opportunities for further exploration and learning. Whether you’re a seasoned developer or just getting started with Node.js, there’s always something new to discover and learn. By staying curious, experimenting with new ideas and technologies, and actively participating in the vibrant Node.js community, developers can continue to grow their skills and expertise and stay at the forefront of backend development innovation.